PowerShell Script to check for AAD Apps with expiring ClientSecrets and Certificates
Hi all,
I guess we all wrote Applications or Scripts that use AAD Applications and used ClientSecrets or Certificates to Authenticate. But what will happen when the Certificate or ClientSecret will expire? Simple - the Application won't work anymore, because the Authentication will fail.
So i searched for a way to check if the ClientSecret or Certificate will soon expire.
Let me show an Application that has multiple ClientSecrets and Certificates.
Here's the Overview of an AAD Application in the Azure AD Portal https://aad.portal.azure.com/#blade/Microsoft_AAD_IAM/ActiveDirectoryMenuBlade/RegisteredApps
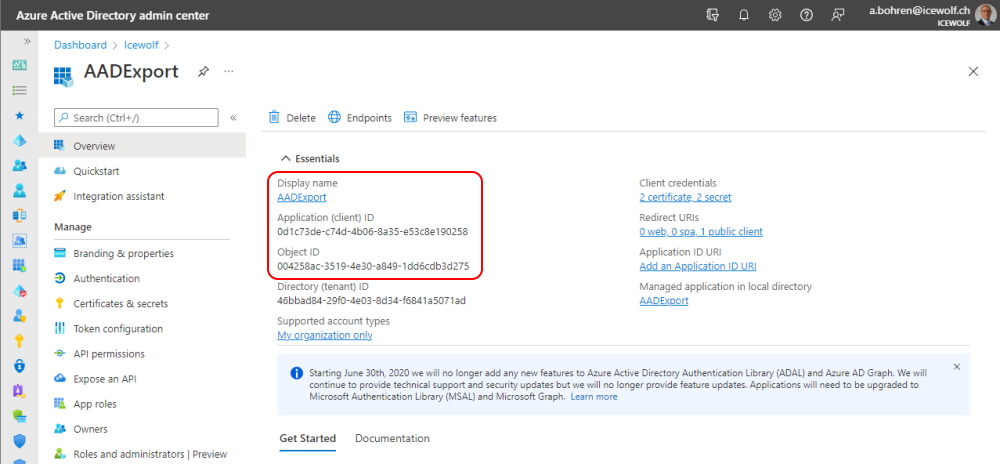
There are two ClientSecrets
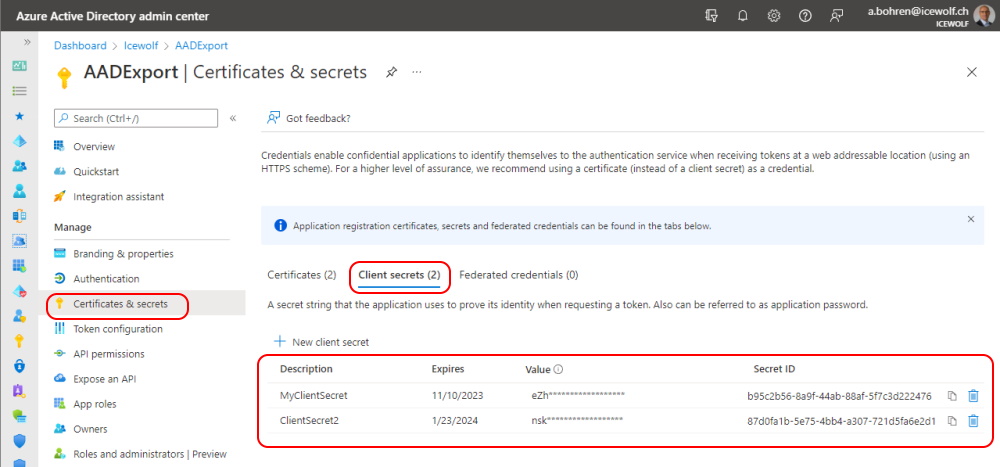
There are two Certificates
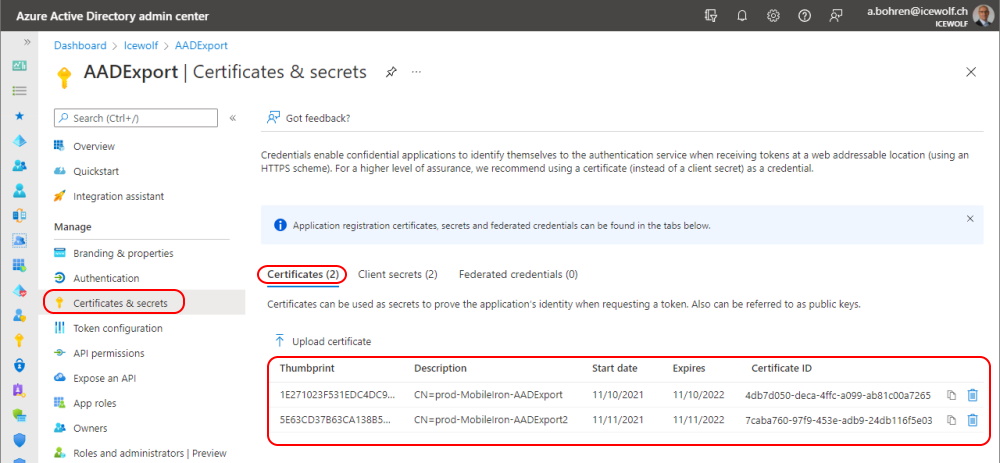
Make sure there is an Owner of the Application with an Emailaddress
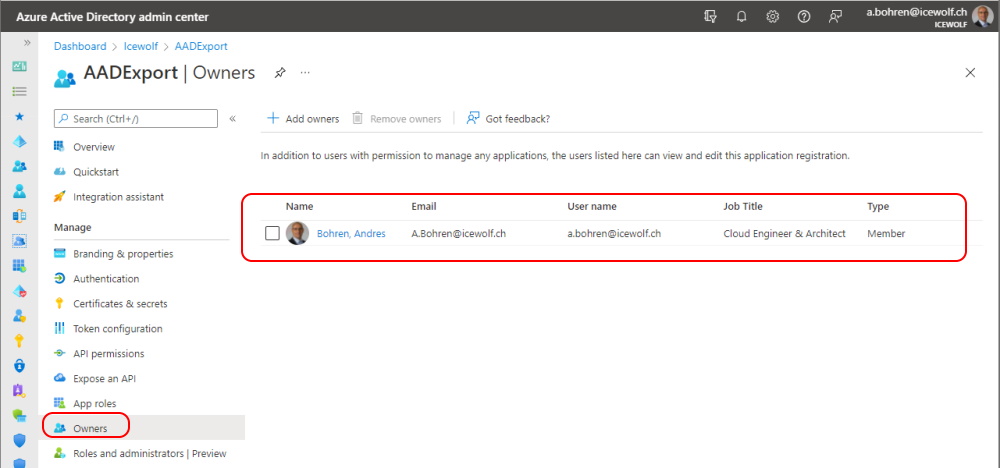
All you need is the AzureAD PowerShell Module
Connect-AzureAD
$AzureADApp = Get-AzureADApplication -ObjectId 004258ac-3519-4e30-a849-1dd6cdb3d275
$AzureADApp = Get-AzureADApplication -ObjectId 004258ac-3519-4e30-a849-1dd6cdb3d275
$AzureADApp | fl ObjectId, AppID, DisplayName, KeyCredentials, PasswordCredentials
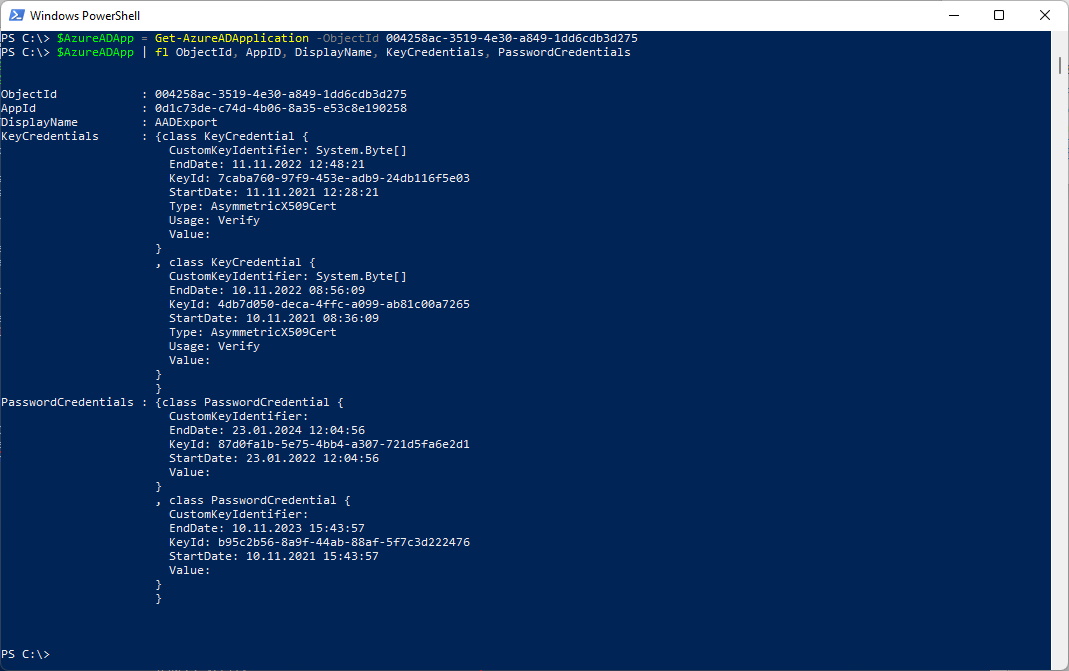
You can also find the Application Owner with PowerShell
#App Owner
Get-AzureADApplicationOwner -ObjectId e09b2538-a844-4889-9af9-78405bb289f7 | Format-List UserPrincipalName, Mail
Get-AzureADApplicationOwner -ObjectId e09b2538-a844-4889-9af9-78405bb289f7 | Format-List UserPrincipalName, Mail

We have all we need to create a simple Script, that will send out Notifications to the Owners if theyr ClientSecrets or Certifications are about to Expire. The Script is quite Simple and can be improved for sure. It's just a starting point...
It can be easy ported to a Runbook in Azure Automation
###############################################################################
# Check AzureAD Apps for Certificates and Clientsecrets that are about to expire
# 2022.02.28 Andres Bohren
###############################################################################
# Interactive Script
# Modules: AzureAD
# Needed Azure AD Role: Directory readers
###############################################################################
#Send Mail Function
###############################################################################
Function Send-EmailNotification{
Param(
[parameter(Mandatory=$true)][String]$to,
[parameter(Mandatory=$true)][String]$Subject,
[parameter(Mandatory=$true)][String]$Body
)
$from = "admin@runbook.icewolf.ch"
#$to = "a.bohren@icewolf.ch"
#$subject = "Azure AD App"
$smtpserver = "icewolfch.mail.protection.outlook.com"
$port = "25"
#$Body = $HTMLBody
#Send-MailMessage -From $From -To $to -Subject $Subject -Body $Body -BodyAsHtml -SmtpServer $SMTPServer -port $port
Send-MailMessage -From $From -To $to -Subject $Subject -Body $Body -SmtpServer $SMTPServer -port $port
}
#Connect AzureAD
Connect-AzureAD
#Get Azure AD Apps
$AzureADApps =Get-AzureADApplication -All $true
#Expiration Comparsion Date
$ExpirationDate = (Get-Date).Adddays(+60)
#Loop through Azure AD Apps
Foreach ($App in $AzureADApps)
{
#Write DisplayName and AppID
Write-Host "$($App.DisplayName) > $($App.AppID)" -ForegroundColor Green
#Show App Owner UPN/Mail
$AppObjectID = $App.ObjectID
$AppOwners = Get-AzureADApplicationOwner -ObjectId $AppObjectID
Foreach ($AppOwner in $AppOwners)
{
Write-Host "AppOwnerUPN: $($AppOwner.UserPrincipalName) > Mail: $($AppOwner.Mail)"
}
#Certificates
If ($Null -ne $App.KeyCredentials)
{
Foreach ($KeyCredential in $App.KeyCredentials)
{
#Write-Host "$($App.DisplayName)" -ForegroundColor Green
$KeyID = $KeyCredential.KeyID
$StartDate = $KeyCredential.StartDate
$EndDate = $KeyCredential.EndDate
Write-Host "KeyID: $KeyID > StartDate: $StartDate > EndDate: $EndDate"
If ($ExpirationDate -gt $EndDate)
{
Write-Host "Certificate will soon expire" -foregroundColor Yellow
Send-EmailNotification -to $AppOwner.Mail -Subject "$($App.DisplayName) Certificate will Expire" -Body "The Azure AD App Certificate will soon expire `r`nKeyID: $KeyID > StartDate: $StartDate > EndDate: $EndDate"
} else {
#Write-Host "Certificate Enddate: $($KeyCredential.Enddate)"
}
}
}
#ClientSecrets
If ($null -ne $AzureADApp.PasswordCredentials)
{
Foreach ($PasswordCredential in $App.PasswordCredentials)
{
#Write-Host "$($App.DisplayName)" -ForegroundColor Green
$KeyID = $PasswordCredential.KeyID
$StartDate = $PasswordCredential.StartDate
$EndDate = $PasswordCredential.EndDate
Write-Host "KeyID: $KeyID > StartDate: $StartDate > EndDate: $EndDate"
If ($ExpirationDate -gt $EndDate)
{
Write-Host "Client Secret will soon expire" -foregroundColor Yellow
Send-EmailNotification -to $AppOwner.Mail -Subject "$($App.DisplayName) ClientCertificate will Expire" -Body "The Azure AD App ClientSecret will soon expire `r`nKeyID: $KeyID > StartDate: $StartDate > EndDate: $EndDate"
} else {
#Write-Host "Certificate Enddate: $($KeyCredential.Enddate)"
}
}
}
}
# Check AzureAD Apps for Certificates and Clientsecrets that are about to expire
# 2022.02.28 Andres Bohren
###############################################################################
# Interactive Script
# Modules: AzureAD
# Needed Azure AD Role: Directory readers
###############################################################################
#Send Mail Function
###############################################################################
Function Send-EmailNotification{
Param(
[parameter(Mandatory=$true)][String]$to,
[parameter(Mandatory=$true)][String]$Subject,
[parameter(Mandatory=$true)][String]$Body
)
$from = "admin@runbook.icewolf.ch"
#$to = "a.bohren@icewolf.ch"
#$subject = "Azure AD App"
$smtpserver = "icewolfch.mail.protection.outlook.com"
$port = "25"
#$Body = $HTMLBody
#Send-MailMessage -From $From -To $to -Subject $Subject -Body $Body -BodyAsHtml -SmtpServer $SMTPServer -port $port
Send-MailMessage -From $From -To $to -Subject $Subject -Body $Body -SmtpServer $SMTPServer -port $port
}
#Connect AzureAD
Connect-AzureAD
#Get Azure AD Apps
$AzureADApps =Get-AzureADApplication -All $true
#Expiration Comparsion Date
$ExpirationDate = (Get-Date).Adddays(+60)
#Loop through Azure AD Apps
Foreach ($App in $AzureADApps)
{
#Write DisplayName and AppID
Write-Host "$($App.DisplayName) > $($App.AppID)" -ForegroundColor Green
#Show App Owner UPN/Mail
$AppObjectID = $App.ObjectID
$AppOwners = Get-AzureADApplicationOwner -ObjectId $AppObjectID
Foreach ($AppOwner in $AppOwners)
{
Write-Host "AppOwnerUPN: $($AppOwner.UserPrincipalName) > Mail: $($AppOwner.Mail)"
}
#Certificates
If ($Null -ne $App.KeyCredentials)
{
Foreach ($KeyCredential in $App.KeyCredentials)
{
#Write-Host "$($App.DisplayName)" -ForegroundColor Green
$KeyID = $KeyCredential.KeyID
$StartDate = $KeyCredential.StartDate
$EndDate = $KeyCredential.EndDate
Write-Host "KeyID: $KeyID > StartDate: $StartDate > EndDate: $EndDate"
If ($ExpirationDate -gt $EndDate)
{
Write-Host "Certificate will soon expire" -foregroundColor Yellow
Send-EmailNotification -to $AppOwner.Mail -Subject "$($App.DisplayName) Certificate will Expire" -Body "The Azure AD App Certificate will soon expire `r`nKeyID: $KeyID > StartDate: $StartDate > EndDate: $EndDate"
} else {
#Write-Host "Certificate Enddate: $($KeyCredential.Enddate)"
}
}
}
#ClientSecrets
If ($null -ne $AzureADApp.PasswordCredentials)
{
Foreach ($PasswordCredential in $App.PasswordCredentials)
{
#Write-Host "$($App.DisplayName)" -ForegroundColor Green
$KeyID = $PasswordCredential.KeyID
$StartDate = $PasswordCredential.StartDate
$EndDate = $PasswordCredential.EndDate
Write-Host "KeyID: $KeyID > StartDate: $StartDate > EndDate: $EndDate"
If ($ExpirationDate -gt $EndDate)
{
Write-Host "Client Secret will soon expire" -foregroundColor Yellow
Send-EmailNotification -to $AppOwner.Mail -Subject "$($App.DisplayName) ClientCertificate will Expire" -Body "The Azure AD App ClientSecret will soon expire `r`nKeyID: $KeyID > StartDate: $StartDate > EndDate: $EndDate"
} else {
#Write-Host "Certificate Enddate: $($KeyCredential.Enddate)"
}
}
}
}
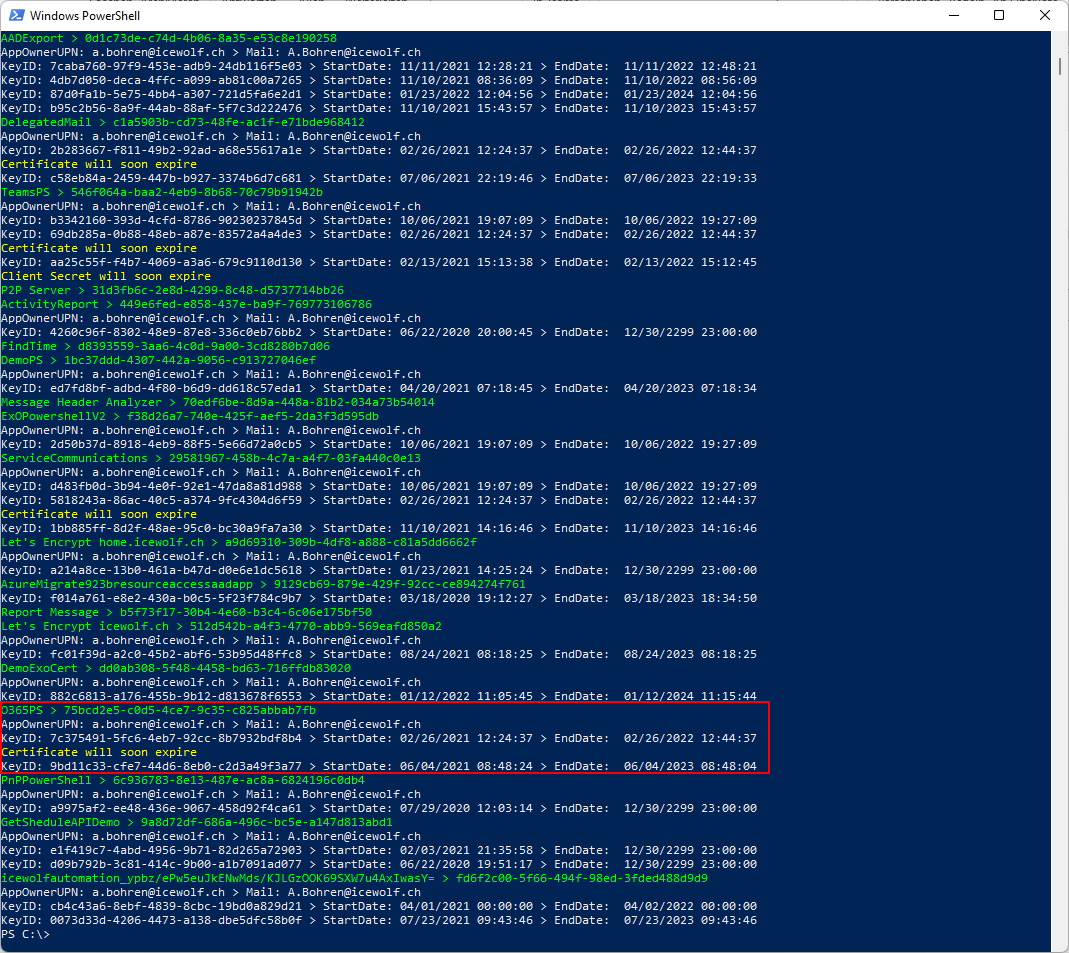
This is the Email generated for the AzureAD App: "O365PS"
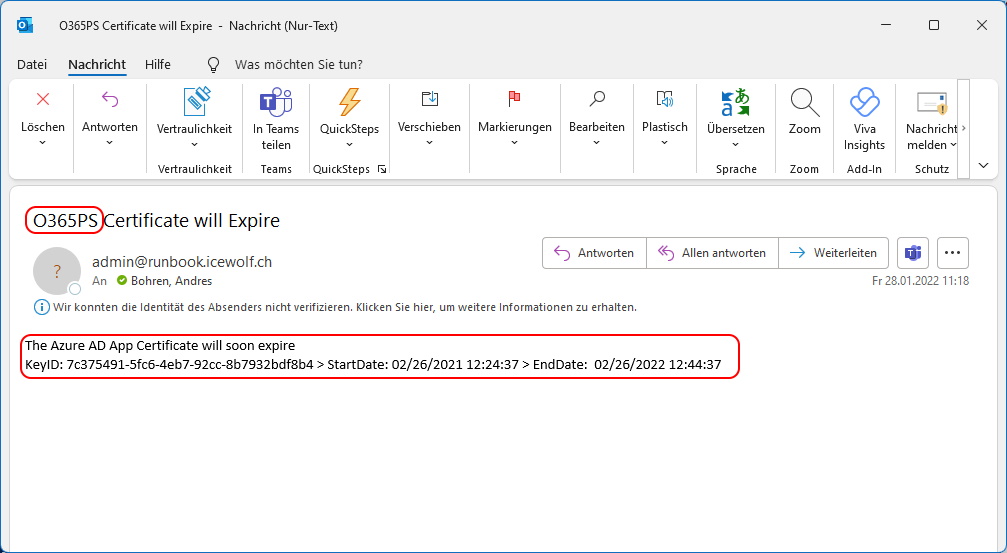
Regards
Andres Bohren
