Simple Example of Sending Mail via Microsoft Graph
Hi All,
I did feel to write a simple PowerShell Script to demonstrate how to Send a Mail via Microsoft Graph.
You need to create an Azure AD Application with the following Permission "Application -> Mail.Send".
Authentication with a SelfSigned Certificate.
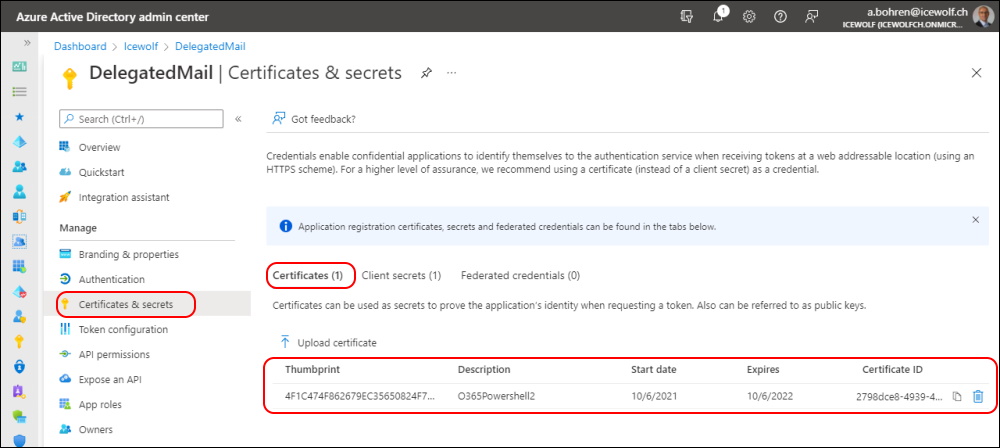
The whole Script is published at my GitHub Repo
###############################################################################
# Limiting application permissions to specific Exchange Online mailboxes
# https://docs.microsoft.com/en-us/graph/auth-limit-mailbox-access
#
# Limit Microsoft Graph Access to specific Exchange Mailboxes
# https://blog.icewolf.ch/archive/2021/02/06/limit-microsoft-graph-access-to-specific-exchange-mailboxes.aspx
###############################################################################
# Limiting application permissions to specific Exchange Online mailboxes
# https://docs.microsoft.com/en-us/graph/auth-limit-mailbox-access
#
# Limit Microsoft Graph Access to specific Exchange Mailboxes
# https://blog.icewolf.ch/archive/2021/02/06/limit-microsoft-graph-access-to-specific-exchange-mailboxes.aspx
###############################################################################
#Mail Enabled Security Group
Get-AzureADGroup -SearchString PostmasterGraphRestriction | Format-Table DisplayName, ObjectId, SecurityEnabled, MailEnabled, Mail
New-ApplicationAccessPolicy -AccessRight RestrictAccess -AppId c1a5903b-cd73-48fe-ac1f-e71bde968412 -PolicyScopeGroupId PostmasterGraphRestriction@icewolf.ch -Description "Restrict this app to members of this Group"
New-ApplicationAccessPolicy -AccessRight RestrictAccess -AppId c1a5903b-cd73-48fe-ac1f-e71bde968412 -PolicyScopeGroupId PostmasterGraphRestriction@icewolf.ch -Description "Restrict this app to members of this Group"
Get-ApplicationAccessPolicy
Test-ApplicationAccessPolicy -AppId c1a5903b-cd73-48fe-ac1f-e71bde968412 -Identity postmaster@icewolf.ch
Test-ApplicationAccessPolicy -AppId c1a5903b-cd73-48fe-ac1f-e71bde968412 -Identity SharedMBX@icewolf.ch
Test-ApplicationAccessPolicy -AppId c1a5903b-cd73-48fe-ac1f-e71bde968412 -Identity postmaster@icewolf.ch
Test-ApplicationAccessPolicy -AppId c1a5903b-cd73-48fe-ac1f-e71bde968412 -Identity SharedMBX@icewolf.ch
For aquiring the Access Token i use the PowerShell Module MSAL.PS
#Import PS Module
Import-Module MSAL.PS
Import-Module MSAL.PS
###############################################################################
# Get AccessToken with MSAL
###############################################################################
#Variables
$TenantId = "icewolfch.onmicrosoft.com"
$AppID = "c1a5903b-cd73-48fe-ac1f-e71bde968412" #DelegatedMail
$CertificateThumbprint = "4F1C474F862679EC35650824F73903041E1E5742" #O365Powershell2.cer
$RedirectUri = "https://login.microsoftonline.com/common/oauth2/nativeclient"
$Scope = "https://graph.microsoft.com/.default"
#Authenticate with Certificate
$Certificate = Get-ChildItem -Path cert:\CurrentUser\my\$CertificateThumbprint
$Token = Get-MsalToken -ClientId $AppID -ClientCertificate $Certificate -TenantId $TenantID -Scope $Scope -RedirectUri $RedirectUri
$AccessToken = $Token.AccessToken
# Get AccessToken with MSAL
###############################################################################
#Variables
$TenantId = "icewolfch.onmicrosoft.com"
$AppID = "c1a5903b-cd73-48fe-ac1f-e71bde968412" #DelegatedMail
$CertificateThumbprint = "4F1C474F862679EC35650824F73903041E1E5742" #O365Powershell2.cer
$RedirectUri = "https://login.microsoftonline.com/common/oauth2/nativeclient"
$Scope = "https://graph.microsoft.com/.default"
#Authenticate with Certificate
$Certificate = Get-ChildItem -Path cert:\CurrentUser\my\$CertificateThumbprint
$Token = Get-MsalToken -ClientId $AppID -ClientCertificate $Certificate -TenantId $TenantID -Scope $Scope -RedirectUri $RedirectUri
$AccessToken = $Token.AccessToken
Now you have aquired the Access Token and this will be used for the Authentication on the HTTP POST Request to send a Mail
###############################################################################
# Sends Mail via Microsoft Graph API
# https://docs.microsoft.com/en-us/graph/api/user-sendmail?view=graph-rest-1.0&tabs=http
###############################################################################
#Delegated (work or school account) Mail.Send
#Delegated (personal Microsoft account) Mail.Send
#Application Mail.Send
#Create HTML Body
[string]$body = @"
<html>
<head>
<style>
p {
text-align: Left;
color: green;
font-size: 12px;
font-family: Arial
}
table, th, td {
border: 1px solid;
font-size: 12px;
font-family: Arial
}
</style>
</head>
<body>
<h3>HTML Header</h3>
<p>the quick brown fox jumps over the lazy dog</p>
</body>
</html>
"@
# Sends Mail via Microsoft Graph API
# https://docs.microsoft.com/en-us/graph/api/user-sendmail?view=graph-rest-1.0&tabs=http
###############################################################################
#Delegated (work or school account) Mail.Send
#Delegated (personal Microsoft account) Mail.Send
#Application Mail.Send
#Create HTML Body
[string]$body = @"
<html>
<head>
<style>
p {
text-align: Left;
color: green;
font-size: 12px;
font-family: Arial
}
table, th, td {
border: 1px solid;
font-size: 12px;
font-family: Arial
}
</style>
</head>
<body>
<h3>HTML Header</h3>
<p>the quick brown fox jumps over the lazy dog</p>
</body>
</html>
"@
Now we can send the Acutal Mail
$Mailbox = "postmaster@icewolf.ch"
$URI = "https://graph.microsoft.com/v1.0/users/$Mailbox/sendMail"
$ContentType = "application/json"
$Headers = @{"Authorization" = "Bearer "+ $AccessToken}
$Body = @"
{
"message": {
"subject": "Microsoft Graph API Mail DEMO",
"body": {
"contentType": "HTML",
"content": "$Body"
},
"toRecipients": [
{
"emailAddress": {
"address": "a.bohren@icewolf.ch"
}
}
]
}
}
"@
#Send Actual Mail
$result = Invoke-RestMethod -Method "POST" -Uri $uri -Body $Body -Headers $Headers -ContentType $ContentType
If ($null -ne $result)
{
Write-Host "Mail has been sucessufully sent"
}
$URI = "https://graph.microsoft.com/v1.0/users/$Mailbox/sendMail"
$ContentType = "application/json"
$Headers = @{"Authorization" = "Bearer "+ $AccessToken}
$Body = @"
{
"message": {
"subject": "Microsoft Graph API Mail DEMO",
"body": {
"contentType": "HTML",
"content": "$Body"
},
"toRecipients": [
{
"emailAddress": {
"address": "a.bohren@icewolf.ch"
}
}
]
}
}
"@
#Send Actual Mail
$result = Invoke-RestMethod -Method "POST" -Uri $uri -Body $Body -Headers $Headers -ContentType $ContentType
If ($null -ne $result)
{
Write-Host "Mail has been sucessufully sent"
}
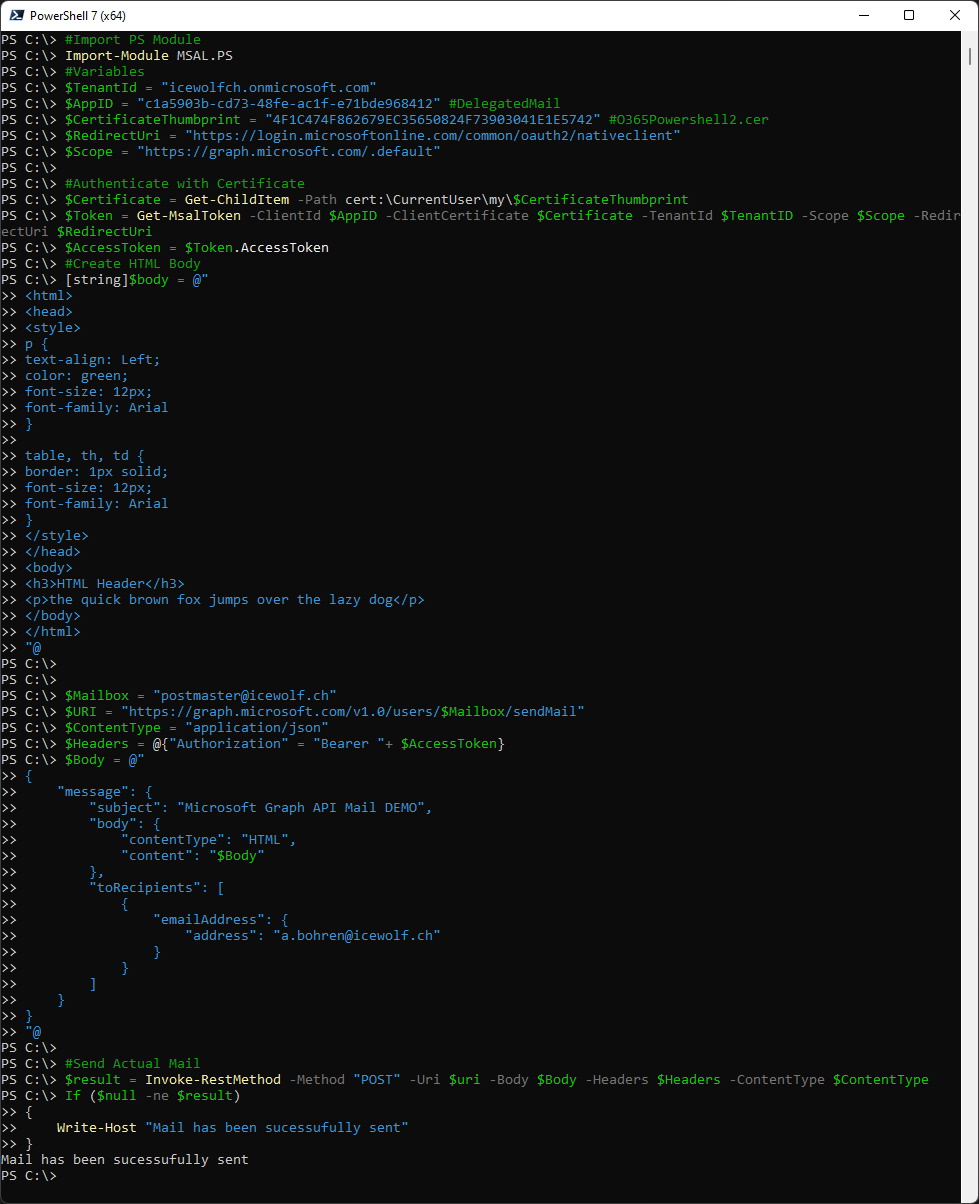
The HTML Mail looks like this
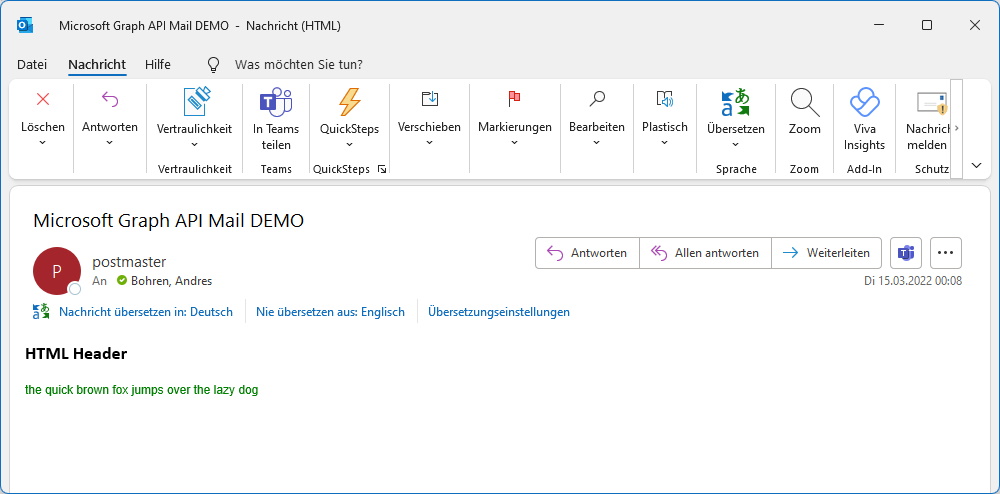
Regards
Andres Bohren
