How to Manage PowerShell 5 and 7 Modules on Azure Automation
Hi All,
As you might already know, i am a big Fan of Azure Automation.
Recently i have used "Managed Identity with Exchange Online on Azure Automation".
Yesterday there was a Release of "Microsoft.Graph PowerShell Module 1.17.0" and the Question of how to update the PowerShell Modules on Azure Automate arises once again.
Basically i've covered that already in a Blog Post earlyer this Year "Update Modules on Azure Automation with AZ PowerShell".
But i think i have improved the Script a little bit. And finally i explain how to Manage the PowerShell 7 Modules on Azure Automate.
Connect-AzAccount
#Get Automation Account
Get-AzAutomationAccount
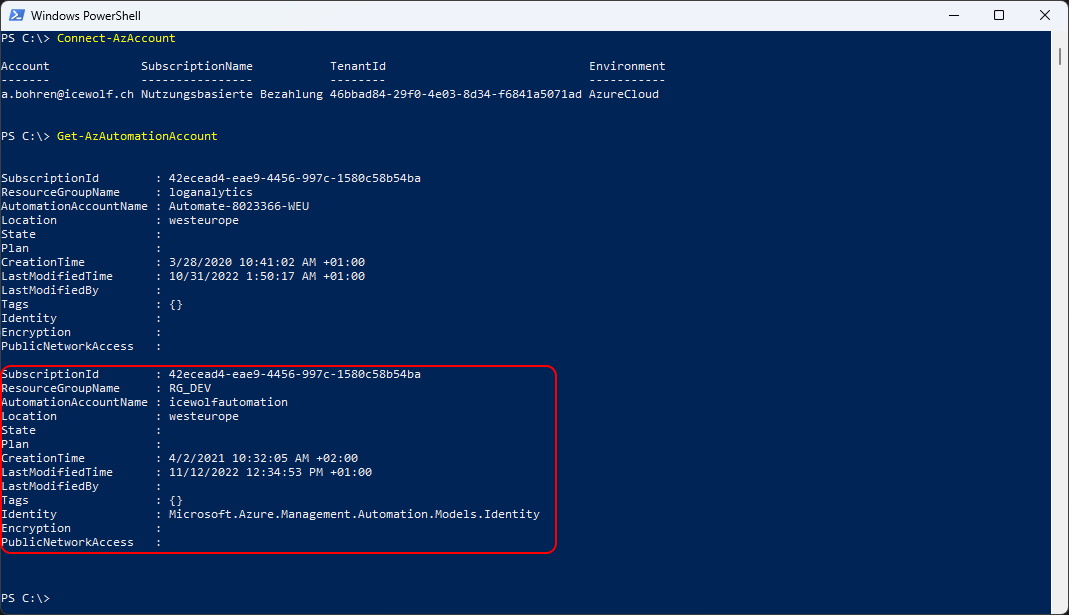
#Get Modules
$accountName = 'icewolfautomation'
$rgName = 'RG_DEV'
$ModuleName = "Microsoft.Graph"
$Modules = Get-AzAutomationModule -AutomationAccountName $accountName -ResourceGroupName $rgName | Where-Object {$_.Name -match "$ModuleName"}
$Modules
$accountName = 'icewolfautomation'
$rgName = 'RG_DEV'
$ModuleName = "Microsoft.Graph"
$Modules = Get-AzAutomationModule -AutomationAccountName $accountName -ResourceGroupName $rgName | Where-Object {$_.Name -match "$ModuleName"}
$Modules
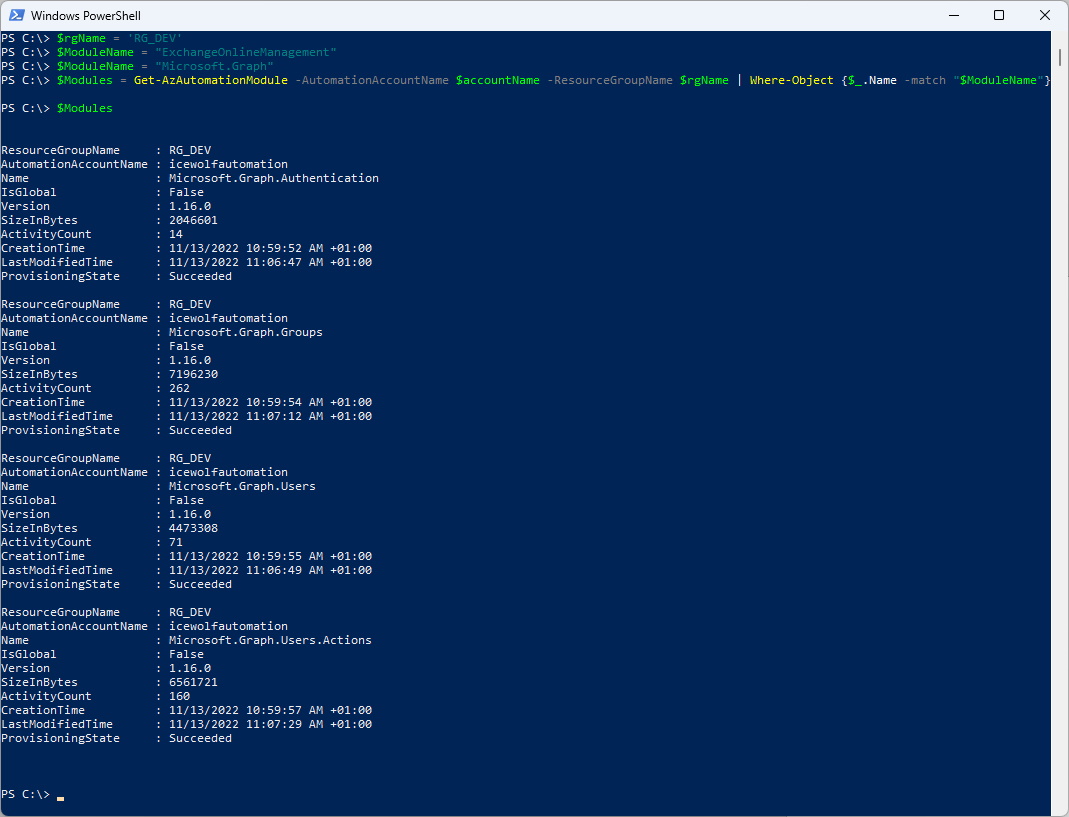
That's the same List as from the Azure Portal
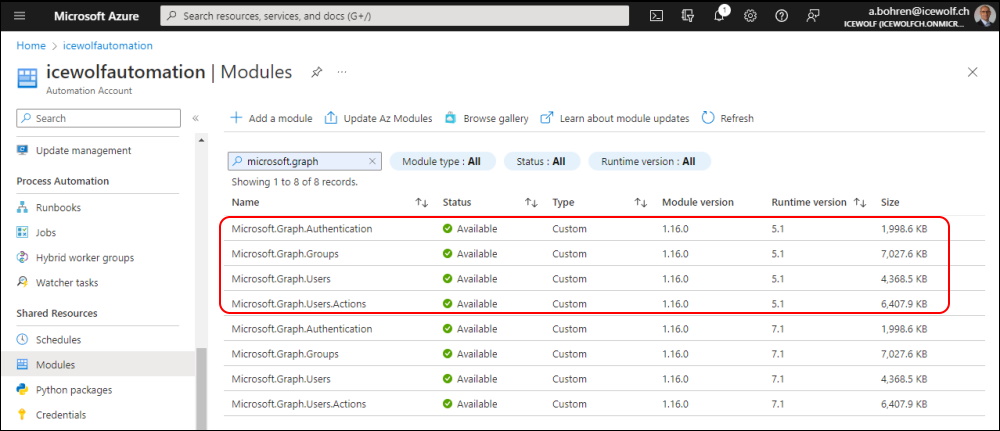
#Remove Module
#For Microsoft.Graph it is important that Microsoft.Graph.Authentication is uninstalled last due to dependencys
Foreach ($Module in $Modules)
{
If ($Module.Name -ne "Microsoft.Graph.Authentication")
{
$ModuleName = $Module.Name
Write-Host "Remove ModuleName: $ModuleName"
Remove-AzAutomationModule -AutomationAccountName $accountName -Name $ModuleName -ResourceGroupName $rgName -Confirm:$False -Force
}
}
$ModuleName = "Microsoft.Graph.Authentication"
Write-Host "Remove ModuleName: $ModuleName"
Remove-AzAutomationModule -AutomationAccountName $accountName -Name $ModuleName -ResourceGroupName $rgName -Confirm:$False -Force
#Add Module
#For Microsoft.Graph it is important that Microsoft.Graph.Authentication is installed first due to dependencys
$ModuleName = "Microsoft.Graph.Authentication"
$moduleVersion = "1.17.0"
New-AzAutomationModule -AutomationAccountName $accountName -ResourceGroupName $rgName -Name $moduleName -ContentLinkUri "https://www.powershellgallery.com/api/v2/package/$moduleName/$moduleVersion"
#For Microsoft.Graph it is important that Microsoft.Graph.Authentication is uninstalled last due to dependencys
Foreach ($Module in $Modules)
{
If ($Module.Name -ne "Microsoft.Graph.Authentication")
{
$ModuleName = $Module.Name
Write-Host "Remove ModuleName: $ModuleName"
Remove-AzAutomationModule -AutomationAccountName $accountName -Name $ModuleName -ResourceGroupName $rgName -Confirm:$False -Force
}
}
$ModuleName = "Microsoft.Graph.Authentication"
Write-Host "Remove ModuleName: $ModuleName"
Remove-AzAutomationModule -AutomationAccountName $accountName -Name $ModuleName -ResourceGroupName $rgName -Confirm:$False -Force
#Add Module
#For Microsoft.Graph it is important that Microsoft.Graph.Authentication is installed first due to dependencys
$ModuleName = "Microsoft.Graph.Authentication"
$moduleVersion = "1.17.0"
New-AzAutomationModule -AutomationAccountName $accountName -ResourceGroupName $rgName -Name $moduleName -ContentLinkUri "https://www.powershellgallery.com/api/v2/package/$moduleName/$moduleVersion"
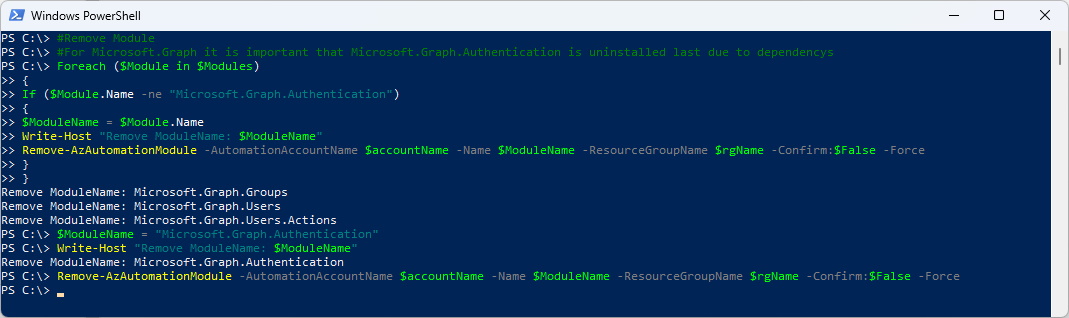
The PowerShell 5 Modules have been removed.
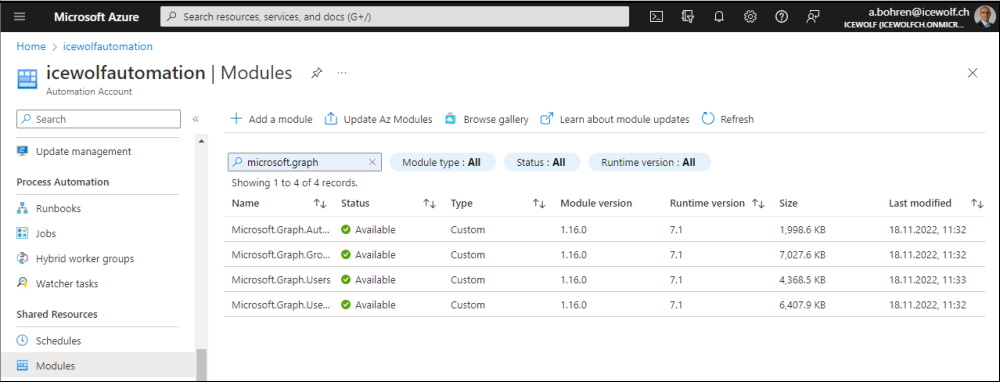
#Add Module
#For Microsoft.Graph it is important that Microsoft.Graph.Authentication is installed first due to dependencys
$ModuleName = "Microsoft.Graph.Authentication"
$moduleVersion = "1.17.0"
New-AzAutomationModule -AutomationAccountName $accountName -ResourceGroupName $rgName -Name $moduleName -ContentLinkUri "https://www.powershellgallery.com/api/v2/package/$moduleName/$moduleVersion"
#Wait until Module is installed
Do {
$Module = Get-AzAutomationModule -AutomationAccountName $accountName -ResourceGroupName $rgName | Where-Object {$_.Name -match "$ModuleName"}
Write-Host "State: $($Module.ProvisioningState) > Check again in 15 Seconds"
Start-Sleep -Seconds 15
} until ($Module.ProvisioningState -eq "Succeeded")
#Install the Rest of the Modules
$Modules = @()
$Modules += "Microsoft.Graph.Users"
$Modules += "Microsoft.Graph.Users.Actions"
$Modules += "Microsoft.Graph.Groups"
$moduleVersion = "1.17.0"
Foreach ($ModuleName in $Modules)
{
Write-Host "ModuleName: $ModuleName"
New-AzAutomationModule -AutomationAccountName $accountName -ResourceGroupName $rgName -Name $moduleName -ContentLinkUri "https://www.powershellgallery.com/api/v2/package/$moduleName/$moduleVersion"
}
#For Microsoft.Graph it is important that Microsoft.Graph.Authentication is installed first due to dependencys
$ModuleName = "Microsoft.Graph.Authentication"
$moduleVersion = "1.17.0"
New-AzAutomationModule -AutomationAccountName $accountName -ResourceGroupName $rgName -Name $moduleName -ContentLinkUri "https://www.powershellgallery.com/api/v2/package/$moduleName/$moduleVersion"
#Wait until Module is installed
Do {
$Module = Get-AzAutomationModule -AutomationAccountName $accountName -ResourceGroupName $rgName | Where-Object {$_.Name -match "$ModuleName"}
Write-Host "State: $($Module.ProvisioningState) > Check again in 15 Seconds"
Start-Sleep -Seconds 15
} until ($Module.ProvisioningState -eq "Succeeded")
#Install the Rest of the Modules
$Modules = @()
$Modules += "Microsoft.Graph.Users"
$Modules += "Microsoft.Graph.Users.Actions"
$Modules += "Microsoft.Graph.Groups"
$moduleVersion = "1.17.0"
Foreach ($ModuleName in $Modules)
{
Write-Host "ModuleName: $ModuleName"
New-AzAutomationModule -AutomationAccountName $accountName -ResourceGroupName $rgName -Name $moduleName -ContentLinkUri "https://www.powershellgallery.com/api/v2/package/$moduleName/$moduleVersion"
}
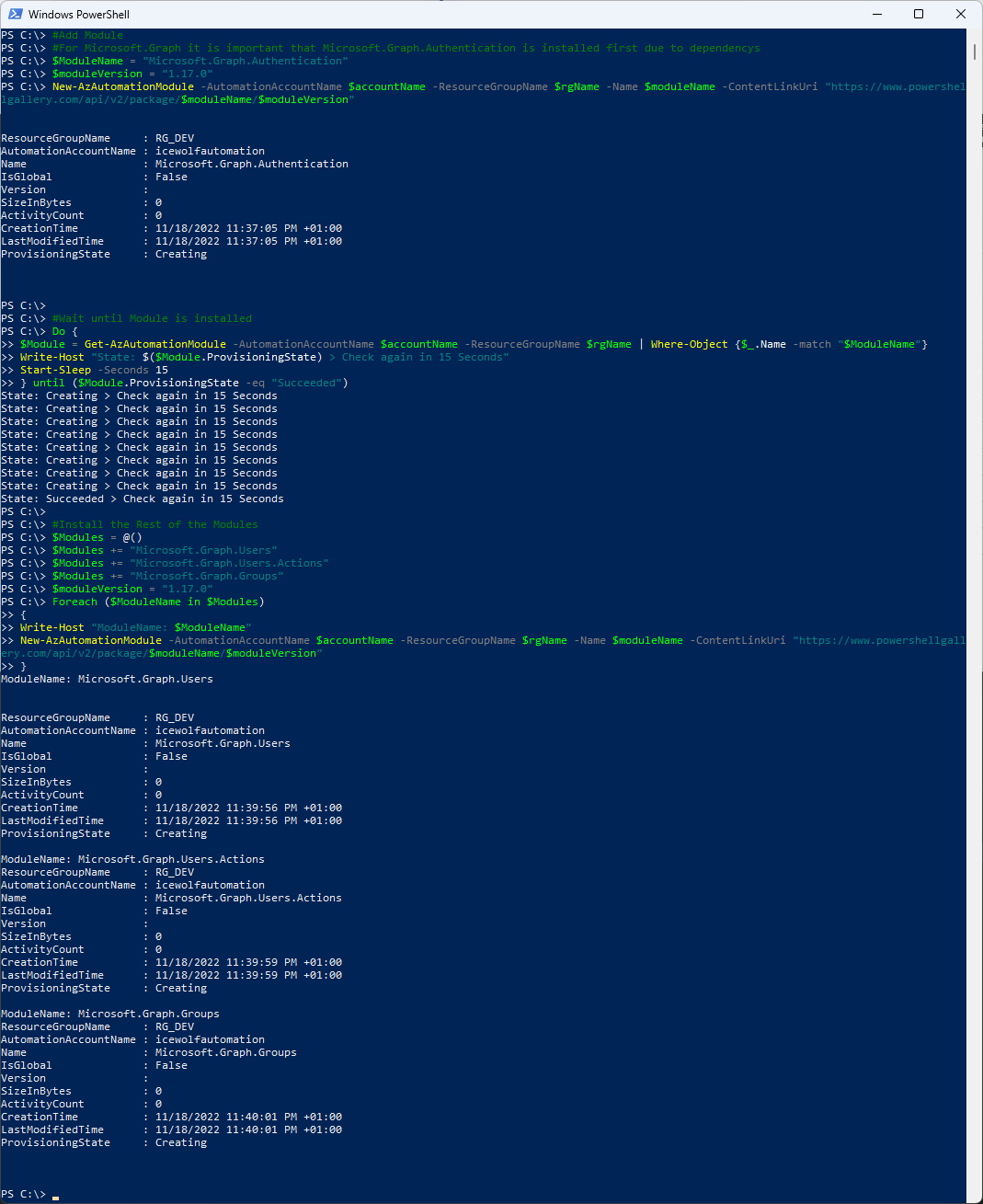
The Microsoft.Graph.Authentication will be installed and after the ProvisioningState is succeeded the Rest of the Modules can be installed and the dependencys will be fullfilled.
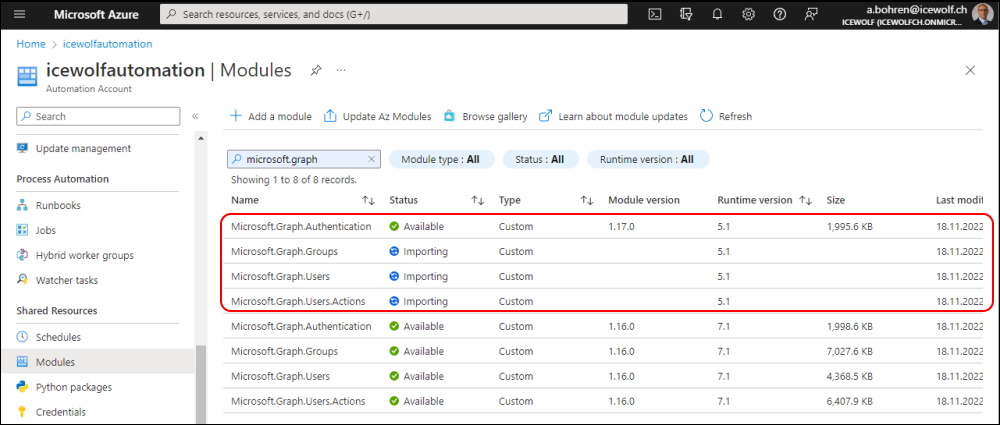
Voila - Upgrade was sucessful
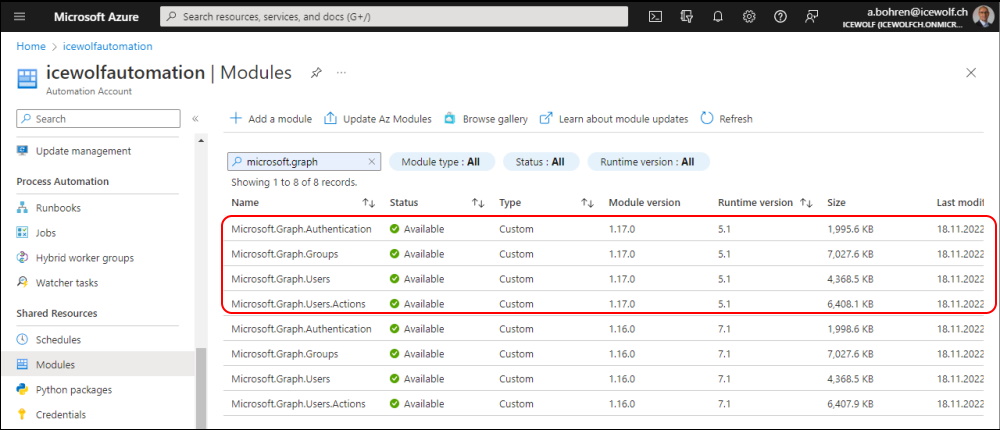
In the Portal you are able to add PowerShell 7 Modules
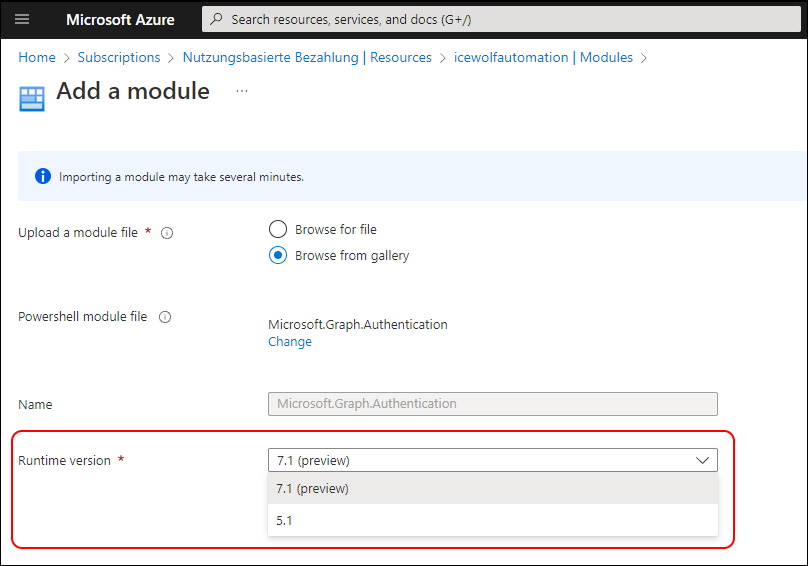
As you can see, there is no Parameter to address PowerShell 7 Modules
New-AzAutomationModule
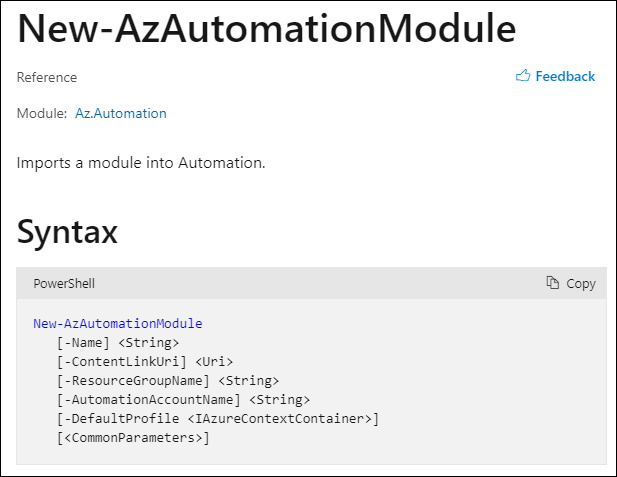
###############################################################################
# GET PS7 Module
###############################################################################
$subscriptionId = "42ecead4-eae9-4456-997c-1580c58b54ba"
$accountName = "icewolfautomation"
$rgName = "RG_DEV"
$ModuleName = "Microsoft.Graph.Authentication"
$Result = Invoke-AzRestMethod `
-Method GET `
-SubscriptionId $subscriptionId `
-ResourceGroupName $rgName `
-ResourceProviderName Microsoft.Automation `
-ResourceType automationAccounts `
-Name $accountName/powershell7Modules/$ModuleName `
-ApiVersion 2019-06-01
($Result.Content | ConvertFrom-Json).Properties
# GET PS7 Module
###############################################################################
$subscriptionId = "42ecead4-eae9-4456-997c-1580c58b54ba"
$accountName = "icewolfautomation"
$rgName = "RG_DEV"
$ModuleName = "Microsoft.Graph.Authentication"
$Result = Invoke-AzRestMethod `
-Method GET `
-SubscriptionId $subscriptionId `
-ResourceGroupName $rgName `
-ResourceProviderName Microsoft.Automation `
-ResourceType automationAccounts `
-Name $accountName/powershell7Modules/$ModuleName `
-ApiVersion 2019-06-01
($Result.Content | ConvertFrom-Json).Properties
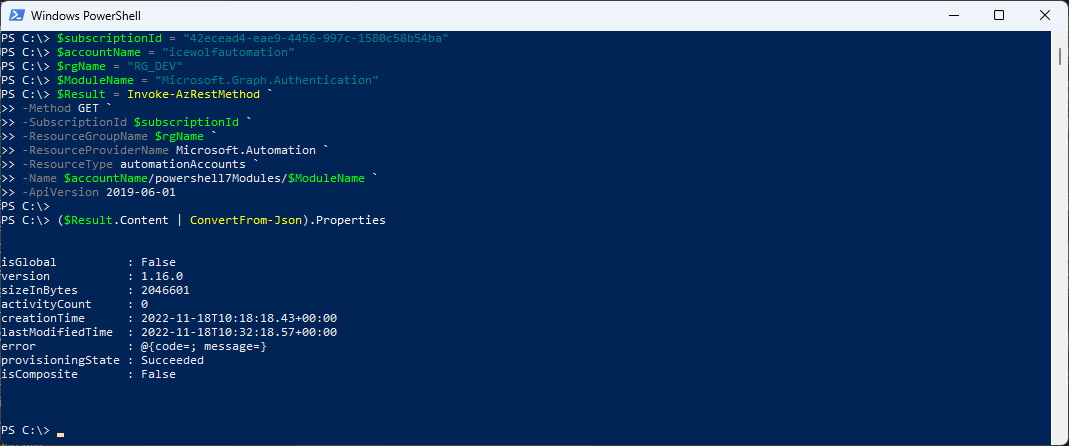
###############################################################################
#Delete PS7 Module
###############################################################################
$subscriptionId = "42ecead4-eae9-4456-997c-1580c58b54ba"
$accountName = "icewolfautomation"
$rgName = "RG_DEV"
$Modules = @()
$Modules += "Microsoft.Graph.Authentication"
$Modules += "Microsoft.Graph.Users"
$Modules += "Microsoft.Graph.Users.Actions"
$Modules += "Microsoft.Graph.Groups"
#For Microsoft.Graph it is important that Microsoft.Graph.Authentication is uninstalled last due to dependencys
Foreach ($ModuleName in $Modules)
{
If ($ModuleName -ne "Microsoft.Graph.Authentication")
{
Write-Host "Remove ModuleName: $ModuleName"
Invoke-AzRestMethod `
-Method DELETE `
-SubscriptionId $subscriptionId `
-ResourceGroupName $rgName `
-ResourceProviderName Microsoft.Automation `
-ResourceType automationAccounts `
-Name $accountName/powershell7Modules/$ModuleName `
-ApiVersion 2019-06-01
}
}
$ModuleName = "Microsoft.Graph.Authentication"
Write-Host "Remove ModuleName: $ModuleName"
Invoke-AzRestMethod `
-Method DELETE `
-SubscriptionId $subscriptionId `
-ResourceGroupName $rgName `
-ResourceProviderName Microsoft.Automation `
-ResourceType automationAccounts `
-Name $accountName/powershell7Modules/$ModuleName `
-ApiVersion 2019-06-01
#Delete PS7 Module
###############################################################################
$subscriptionId = "42ecead4-eae9-4456-997c-1580c58b54ba"
$accountName = "icewolfautomation"
$rgName = "RG_DEV"
$Modules = @()
$Modules += "Microsoft.Graph.Authentication"
$Modules += "Microsoft.Graph.Users"
$Modules += "Microsoft.Graph.Users.Actions"
$Modules += "Microsoft.Graph.Groups"
#For Microsoft.Graph it is important that Microsoft.Graph.Authentication is uninstalled last due to dependencys
Foreach ($ModuleName in $Modules)
{
If ($ModuleName -ne "Microsoft.Graph.Authentication")
{
Write-Host "Remove ModuleName: $ModuleName"
Invoke-AzRestMethod `
-Method DELETE `
-SubscriptionId $subscriptionId `
-ResourceGroupName $rgName `
-ResourceProviderName Microsoft.Automation `
-ResourceType automationAccounts `
-Name $accountName/powershell7Modules/$ModuleName `
-ApiVersion 2019-06-01
}
}
$ModuleName = "Microsoft.Graph.Authentication"
Write-Host "Remove ModuleName: $ModuleName"
Invoke-AzRestMethod `
-Method DELETE `
-SubscriptionId $subscriptionId `
-ResourceGroupName $rgName `
-ResourceProviderName Microsoft.Automation `
-ResourceType automationAccounts `
-Name $accountName/powershell7Modules/$ModuleName `
-ApiVersion 2019-06-01
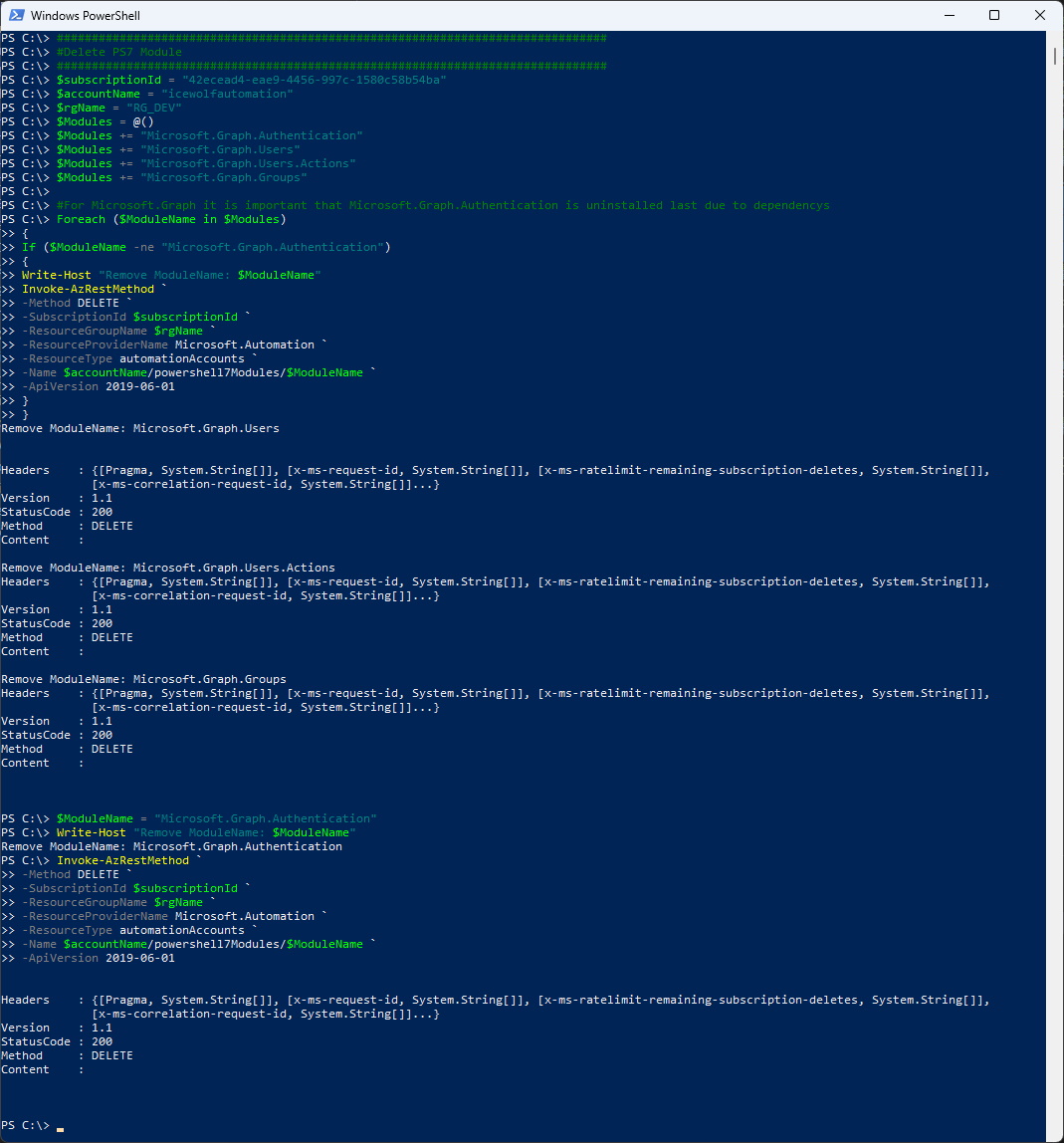
The PowerShell 7 Modules have been removed
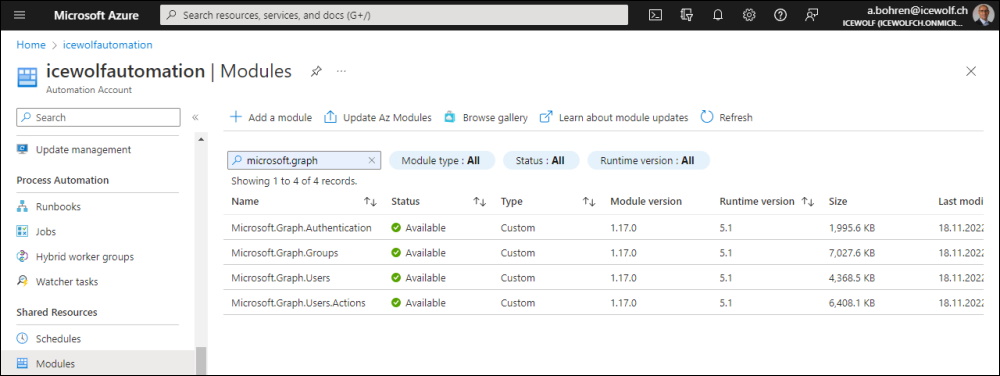
###############################################################################
#Add PS7 Module
###############################################################################
$subscriptionId = "42ecead4-eae9-4456-997c-1580c58b54ba"
$accountName = "icewolfautomation"
$rgName = "RG_DEV"
$ModuleName = "Microsoft.Graph.Authentication"
$moduleVersion = "1.17.0"
#Install Microsoft.Graph.Authentication
$Payload = @"
{"properties":
{"contentLink":
{"uri":"https://www.powershellgallery.com/api/v2/package/$ModuleName/$moduleVersion"}
}
}
"@
Write-Host "Install ModuleName: $ModuleName"
Invoke-AzRestMethod `
-Method PUT `
-SubscriptionId $subscriptionId `
-ResourceGroupName $rgName `
-ResourceProviderName Microsoft.Automation `
-ResourceType automationAccounts `
-Name $accountName/powershell7Modules/$ModuleName `
-ApiVersion 2019-06-01 `
-Payload $Payload
#Wait until Module is installed
Do {
$Result = Invoke-AzRestMethod `
-Method GET `
-SubscriptionId $subscriptionId `
-ResourceGroupName $rgName `
-ResourceProviderName Microsoft.Automation `
-ResourceType automationAccounts `
-Name $accountName/powershell7Modules/$ModuleName `
-ApiVersion 2019-06-01
$ProvisioningState = (($Result.Content | ConvertFrom-Json).Properties).provisioningState
$Module = Get-AzAutomationModule -AutomationAccountName $accountName -ResourceGroupName $rgName | Where-Object {$_.Name -match "$ModuleName"}
Write-Host "State: $ProvisioningState > Check again in 15 Seconds"
Start-Sleep -Seconds 15
} until ($ProvisioningState -eq "Succeeded")
#Add PS7 Module
###############################################################################
$subscriptionId = "42ecead4-eae9-4456-997c-1580c58b54ba"
$accountName = "icewolfautomation"
$rgName = "RG_DEV"
$ModuleName = "Microsoft.Graph.Authentication"
$moduleVersion = "1.17.0"
#Install Microsoft.Graph.Authentication
$Payload = @"
{"properties":
{"contentLink":
{"uri":"https://www.powershellgallery.com/api/v2/package/$ModuleName/$moduleVersion"}
}
}
"@
Write-Host "Install ModuleName: $ModuleName"
Invoke-AzRestMethod `
-Method PUT `
-SubscriptionId $subscriptionId `
-ResourceGroupName $rgName `
-ResourceProviderName Microsoft.Automation `
-ResourceType automationAccounts `
-Name $accountName/powershell7Modules/$ModuleName `
-ApiVersion 2019-06-01 `
-Payload $Payload
#Wait until Module is installed
Do {
$Result = Invoke-AzRestMethod `
-Method GET `
-SubscriptionId $subscriptionId `
-ResourceGroupName $rgName `
-ResourceProviderName Microsoft.Automation `
-ResourceType automationAccounts `
-Name $accountName/powershell7Modules/$ModuleName `
-ApiVersion 2019-06-01
$ProvisioningState = (($Result.Content | ConvertFrom-Json).Properties).provisioningState
$Module = Get-AzAutomationModule -AutomationAccountName $accountName -ResourceGroupName $rgName | Where-Object {$_.Name -match "$ModuleName"}
Write-Host "State: $ProvisioningState > Check again in 15 Seconds"
Start-Sleep -Seconds 15
} until ($ProvisioningState -eq "Succeeded")
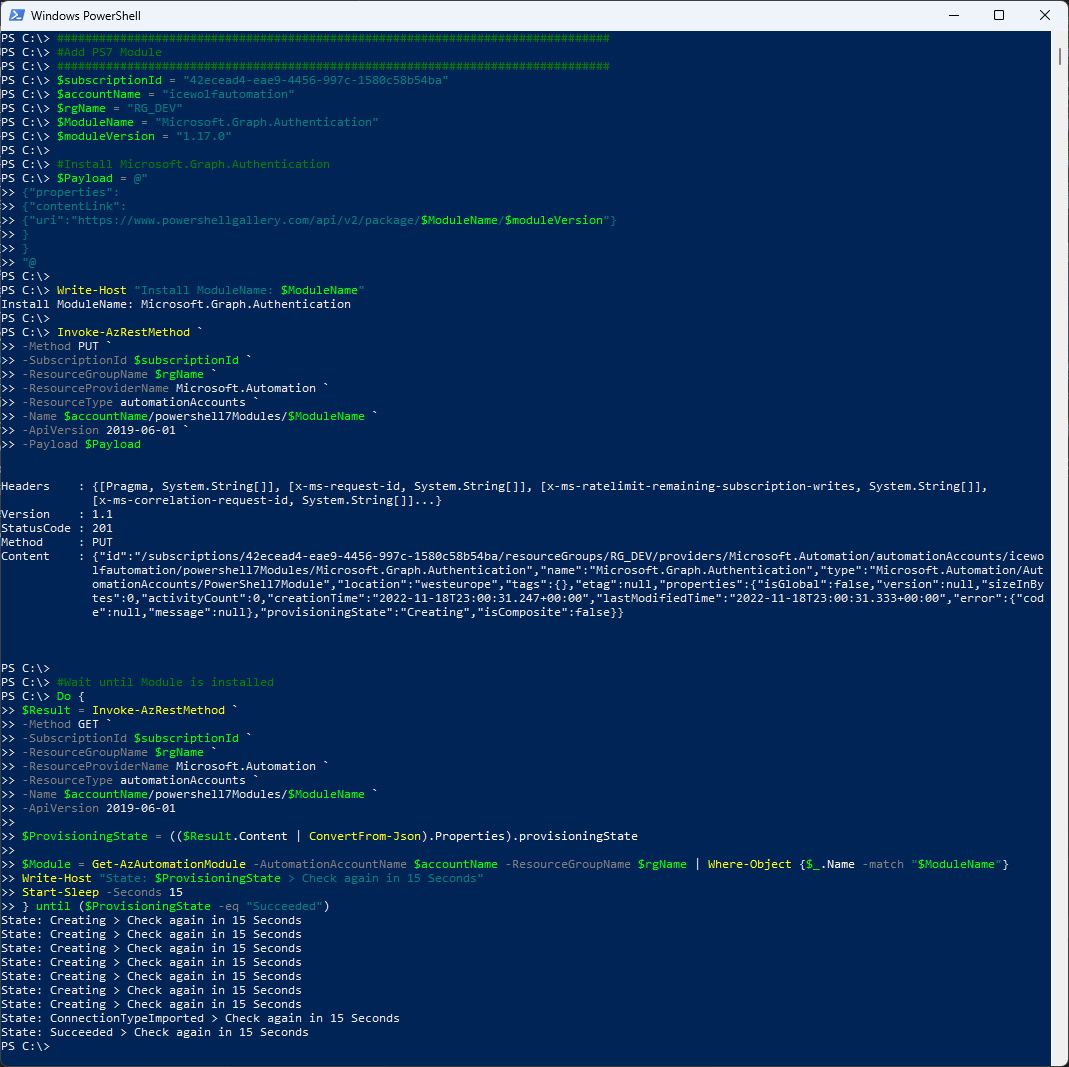
#Now install the Rest of the Modules
$Modules = @()
$Modules += "Microsoft.Graph.Users"
$Modules += "Microsoft.Graph.Users.Actions"
$Modules += "Microsoft.Graph.Groups"
Foreach ($ModuleName in $Modules)
{
$Payload = @"
{"properties":
{"contentLink":
{"uri":"https://www.powershellgallery.com/api/v2/package/$ModuleName/$moduleVersion"}
}
}
"@
Write-Host "Install ModuleName: $ModuleName"
Invoke-AzRestMethod `
-Method PUT `
-SubscriptionId $subscriptionId `
-ResourceGroupName $rgName `
-ResourceProviderName Microsoft.Automation `
-ResourceType automationAccounts `
-Name $accountName/powershell7Modules/$ModuleName `
-ApiVersion 2019-06-01 `
-Payload $Payload
}
$Modules = @()
$Modules += "Microsoft.Graph.Users"
$Modules += "Microsoft.Graph.Users.Actions"
$Modules += "Microsoft.Graph.Groups"
Foreach ($ModuleName in $Modules)
{
$Payload = @"
{"properties":
{"contentLink":
{"uri":"https://www.powershellgallery.com/api/v2/package/$ModuleName/$moduleVersion"}
}
}
"@
Write-Host "Install ModuleName: $ModuleName"
Invoke-AzRestMethod `
-Method PUT `
-SubscriptionId $subscriptionId `
-ResourceGroupName $rgName `
-ResourceProviderName Microsoft.Automation `
-ResourceType automationAccounts `
-Name $accountName/powershell7Modules/$ModuleName `
-ApiVersion 2019-06-01 `
-Payload $Payload
}
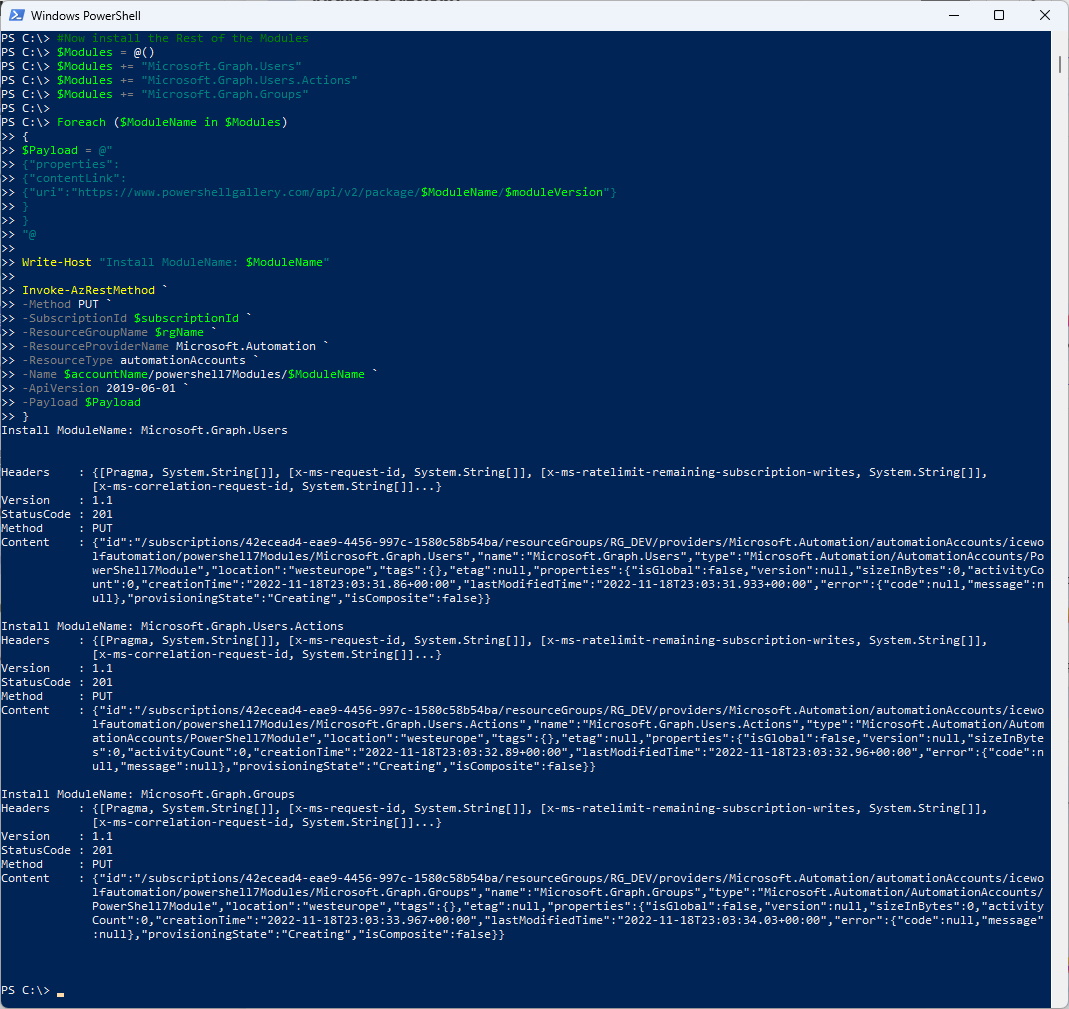
Here as well Microsoft.Graph.Authentication has to be sucessfully installed before the other Microsoft.Graph* Modules can be installed due to Module Dependencys.
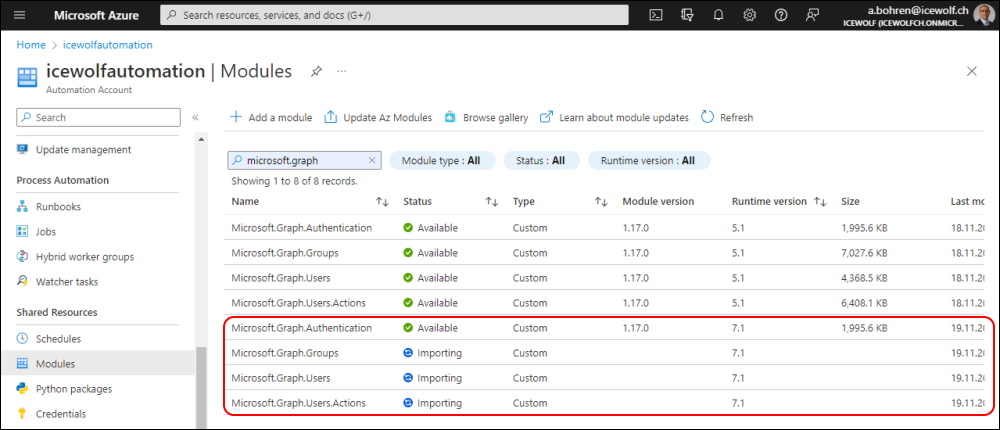
And now all PowerShell 7 Modules on Azure Automate have been updated
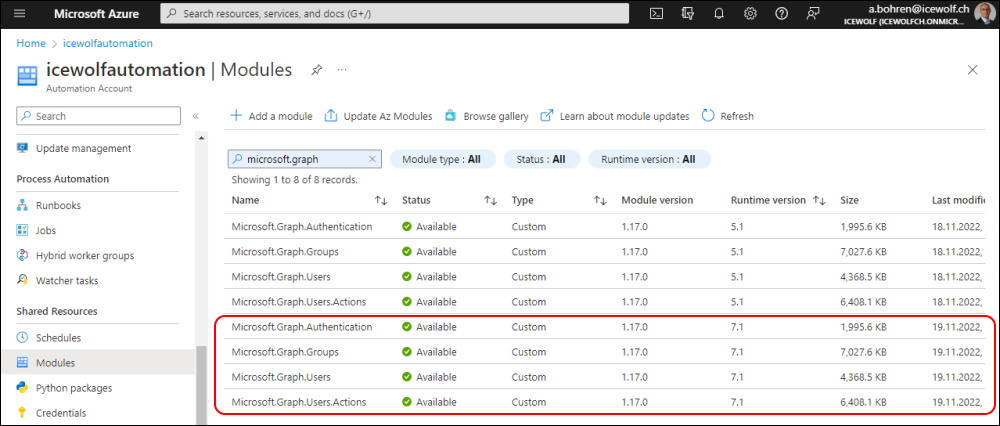
By the Way. These PowerShell Commands are also available on my GitHub Repo
Regards
Andres Bohren
