PowerShell Script to check Entra Apps with expiring ClientSecrets and Certificates
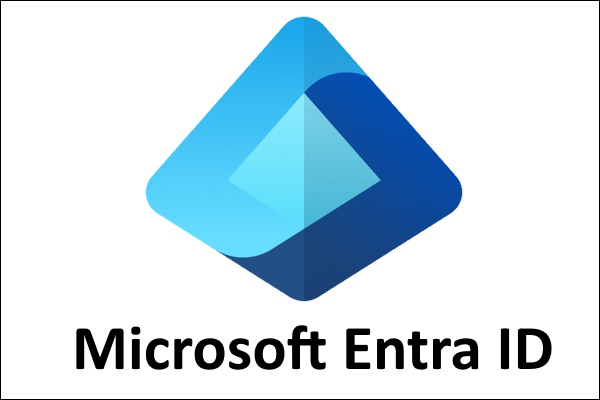
Hi All,
A few years ago, i did write a Script to warn Entra Application Owners about expiring ClientSecrets and Certificates.
The Script has been using the AzureAD PowerShell Module. Time to renew it to Microsoft.Graph
Entra App Registrations
Here is the Code to get the Entra App Registration with Microsoft.Graph PowerShell
#Connect to Microsoft.Graph
Connect-MgGraph -Scopes Application.Read.All -NoWelcome
Let’s have a look at a specific Entra Application
#Get Entra App
$EntraApp = Get-MgApplication -Search "DisplayName:EXORBAC" -ConsistencyLevel "Eventual"
$EntraApp | Format-List
Here is how you get all Entra Applications
#Get All Entra Apps
$EntraApps = Get-MgApplication
$EntraApps
Note: Note the ID and AppID values
But when you use the Parameter -ApplicationId that refers to the ObjectID
#Search for AppID
Get-MgApplication -Search "AppID:0d1c73de-c74d-4b06-8a35-e53c8e190258" -ConsistencyLevel "Eventual"
#Search for Applicaton ObjectId
Get-MgApplication -ApplicationId "004258ac-3519-4e30-a849-1dd6cdb3d275"
Owners
An Application can have multiple Owners
Here is how you get the owners
#Get Owner
$EntraApp = Get-MgApplication -ApplicationId "780ce8e7-9b72-4673-b45a-ee2815f99a44"
$ApplicationObjectId = $EntraApp.Id
$OwnerObject = Get-MgApplicationOwner -ApplicationId $ApplicationObjectId
$Owners = $OwnerObject.AdditionalProperties
$Owners
Certificates
An Application can have multiple Certificates
Here is how you get the Certificates
#Certificates
$EntraApp = Get-MgApplication -ApplicationId "39b4ae73-6234-4ecd-827e-507aa2f25a5b"
$EntraApp.KeyCredentials
$EntraApp.KeyCredentials | Format-List
ClientSecrets
An Application can have mutliple ClientSecrets
Here is how you get the ClientSecrets
#ClientSecret
$EntraApp = Get-MgApplication -ApplicationId "f79a336f-8c16-42a4-b001-21650ad0f479"
$EntraApp.PasswordCredentials
$EntraApp.PasswordCredentials | Format-List
Script
Here is a Script that checks all Entra Applications
- Warns if no Owner is present
- Warns if a Certificate is about to expire
- Warns if a ClientSecret is about to expire
###############################################################################
#Main Script
###############################################################################
Connect-MgGraph -Scopes Application.Read.All -NoWelcome
#Expiration Comparsion Date
$ExpirationDate = (Get-Date).Adddays(+60)
#Get Entra Applications
$EntraApps = Get-MgApplication
#Loop through the Apps
Foreach ($EntraApp in $EntraApps)
{
$DisplayName = $EntraApp.DisplayName
$AppId = $EntraApp.AppId
$ID = $EntraApp.ID
Write-Host "$AppID > $ID > $DisplayName" -ForegroundColor Green
#Get Owner
$OwnerObject = Get-MgApplicationOwner -ApplicationId $ID
$Owners = $OwnerObject.AdditionalProperties
If ($Null -eq $Owners)
{
Write-Host "No Owner found" -ForegroundColor Red
} else {
Foreach ($Owner in $Owners)
{
$UPN = $Owner.userPrincipalName
$Mail = $Owner.mail
Write-Host "OwnerUPN: $UPN > OwnerMail: $Mail"
}
}
#Get Certificates
If ($Null -ne $EntraApp.KeyCredentials)
{
$Certificates = $EntraApp.KeyCredentials
Foreach ($Certificate in $Certificates)
{
$CertDisplayName = $Certificate.DisplayName
$CertStartDate = $Certificate.StartDateTime
$CertEndDate = $Certificate.EndDateTime
Write-Host "Certificate: $CertDisplayName > StartDate: $CertStartDate > EndDate: $CertEndDate"
If ($ExpirationDate -gt $CertEndDate)
{
Write-Host "$CertDisplayName Certificate will soon expire" -foregroundColor Yellow
}
}
}
#Get ClientSecret
If ($Null -ne $EntraApp.PasswordCredentials)
{
$ClientSecrets = $EntraApp.PasswordCredentials
Foreach ($ClientSecret in $ClientSecrets)
{
$DisplayName = $ClientSecret.DisplayName
$StartDate = $ClientSecret.StartDateTime
$EndDate = $ClientSecret.EndDateTime
Write-Host "ClientSecret: $DisplayName > StartDate: $StartDate > EndDate: $EndDate"
If ($ExpirationDate -gt $EndDate)
{
Write-Host "ClientSecret $DisplayName will soon expire" -foregroundColor Yellow
}
}
}
}
Azure Automation
In the next Article, i will show you how to use Azure Automation to do the Checks and inform the Owners.
Regards
Andres Bohren