Use Set/Remove-CsPhoneNumberAssignment in MicrosoftTeams
Hi all,
I hope you all have already seen the Change in M365 Message Center for assigning Teams Phone Number
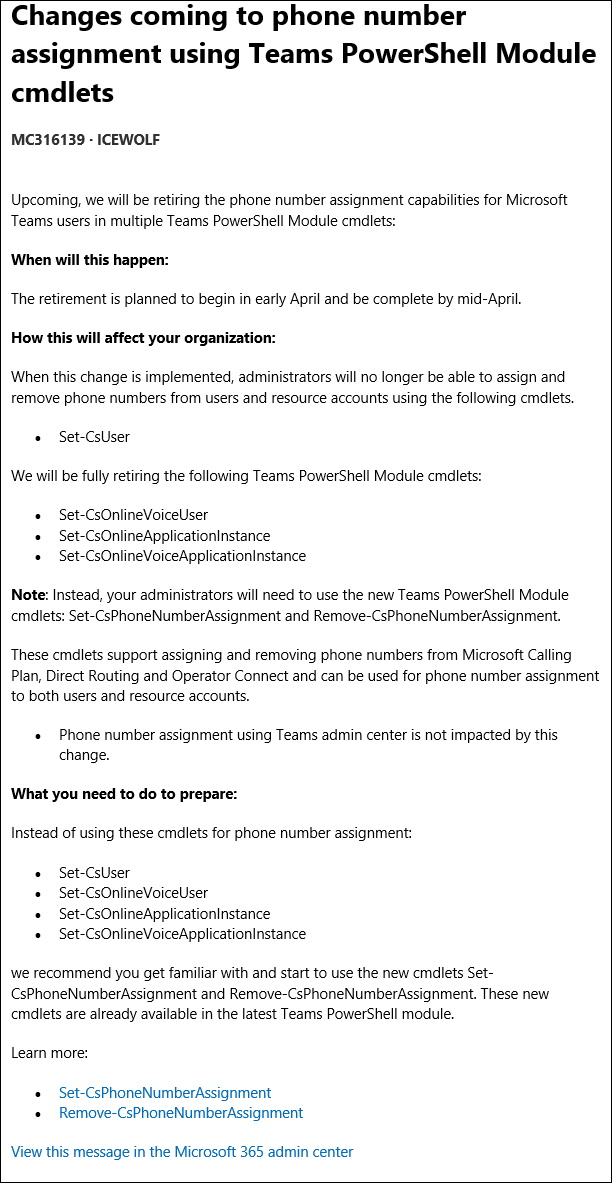
Set-CsPhoneNumberAssignment
https://docs.microsoft.com/en-us/powershell/module/teams/set-csphonenumberassignment?view=teams-ps
https://docs.microsoft.com/en-us/powershell/module/teams/set-csphonenumberassignment?view=teams-ps
Remove-CsPhoneNumberAssignment
https://docs.microsoft.com/en-us/powershell/module/teams/remove-csphonenumberassignment?view=teams-ps
https://docs.microsoft.com/en-us/powershell/module/teams/remove-csphonenumberassignment?view=teams-ps
But there are also other Requirements before adding a Phone Number in Teams:
- The User must have a valid Teams License assigned
- The User must have a Teams Phone (PhoneSystem/MCOEV) License assigned
We can check that with the Microsoft.Graph PowerShell Module
#Get License from User
Connect-MgGraph -Scopes User.ReadWrite.All
$Licenses = Get-MgUserLicenseDetail -UserId a.bohren@icewolf.ch
$Licenses
$Licenses[0].ServicePlans
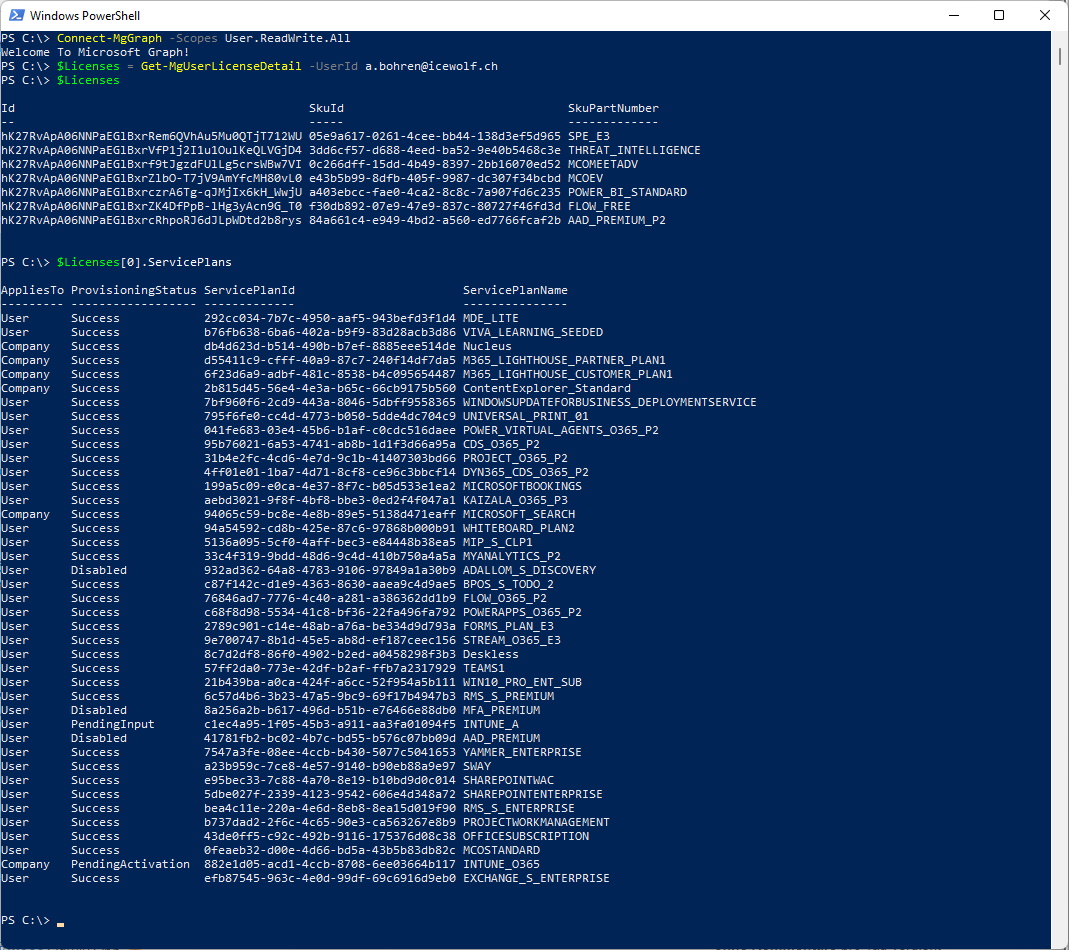
To check the Licenses use this Code
#Teams License
$Licenses | where {$_.ServicePlans.ServicePlanname -match "TEAMS1"}
#PhoneSystem
$Licenses | where {$_.SkuId -eq "e43b5b99-8dfb-405f-9987-dc307f34bcbd"}
$Licenses | where {$_.ServicePlans.ServicePlanname -match "TEAMS1"}
#PhoneSystem
$Licenses | where {$_.SkuId -eq "e43b5b99-8dfb-405f-9987-dc307f34bcbd"}
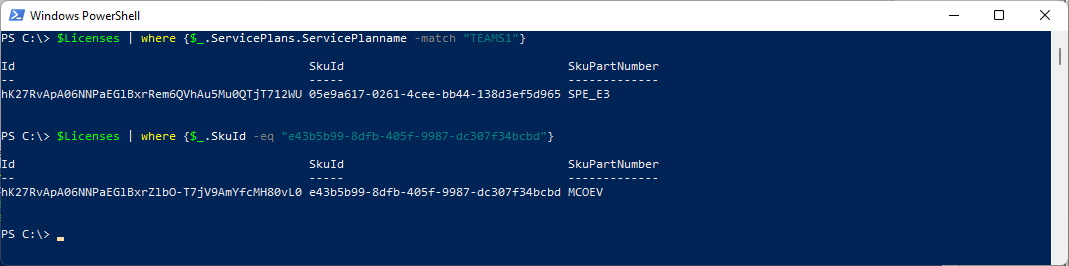
If you need to Add a License use this Code
###############################################################################
# Add PhoneSystem / MCOEV License
###############################################################################
Set-MgUserLicense -UserId m.muster@icewolf.ch -AddLicenses @{SkuId = 'e43b5b99-8dfb-405f-9987-dc307f34bcbd'} -RemoveLicenses @() #PhoneSystem / MCOEV
# Add PhoneSystem / MCOEV License
###############################################################################
Set-MgUserLicense -UserId m.muster@icewolf.ch -AddLicenses @{SkuId = 'e43b5b99-8dfb-405f-9987-dc307f34bcbd'} -RemoveLicenses @() #PhoneSystem / MCOEV

Now let's check the CsUser
###############################################################################
# Get-CsOnlineUser
# https://docs.microsoft.com/en-us/powershell/module/skype/get-csonlineuser?view=skype-ps
###############################################################################
Connect-MicrosoftTeams
Get-CsOnlineUser -Identity m.muster@icewolf.ch | fl EnterpriseVoiceEnabled, *HostingProvider, HostedVoiceMail, *um*, *LineUri
# Get-CsOnlineUser
# https://docs.microsoft.com/en-us/powershell/module/skype/get-csonlineuser?view=skype-ps
###############################################################################
Connect-MicrosoftTeams
Get-CsOnlineUser -Identity m.muster@icewolf.ch | fl EnterpriseVoiceEnabled, *HostingProvider, HostedVoiceMail, *um*, *LineUri
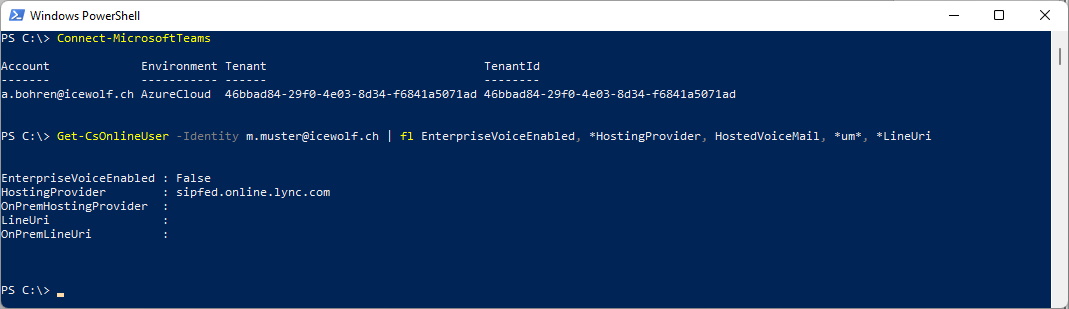
Assign the Number
###############################################################################
# Set-CsPhoneNumberAssignment
# https://docs.microsoft.com/en-us/powershell/module/teams/set-csphonenumberassignment?view=teams-ps
###############################################################################
Set-CsPhoneNumberAssignment -Identity m.muster@icewolf.ch -PhoneNumber +41215553975 -PhoneNumberType DirectRouting
# Set-CsPhoneNumberAssignment
# https://docs.microsoft.com/en-us/powershell/module/teams/set-csphonenumberassignment?view=teams-ps
###############################################################################
Set-CsPhoneNumberAssignment -Identity m.muster@icewolf.ch -PhoneNumber +41215553975 -PhoneNumberType DirectRouting
Get-CsOnlineUser -Identity m.muster@icewolf.ch | fl EnterpriseVoiceEnabled, *HostingProvider, HostedVoiceMail, *um*, *LineUri
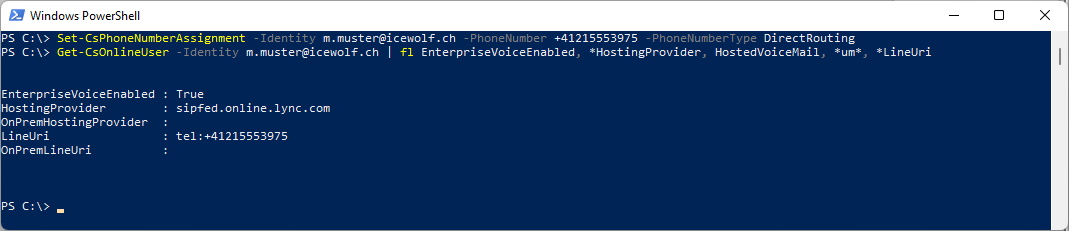
If you want to remove the Number
###############################################################################
# Remove-CsPhoneNumberAssignment
# https://docs.microsoft.com/en-us/powershell/module/teams/remove-csphonenumberassignment?view=teams-ps
###############################################################################
Remove-CsPhoneNumberAssignment -Identity m.muster@icewolf.ch -RemoveAll
# Remove-CsPhoneNumberAssignment
# https://docs.microsoft.com/en-us/powershell/module/teams/remove-csphonenumberassignment?view=teams-ps
###############################################################################
Remove-CsPhoneNumberAssignment -Identity m.muster@icewolf.ch -RemoveAll
Get-CsOnlineUser -Identity m.muster@icewolf.ch | fl EnterpriseVoiceEnabled, *HostingProvider, HostedVoiceMail, *um*, *LineUri
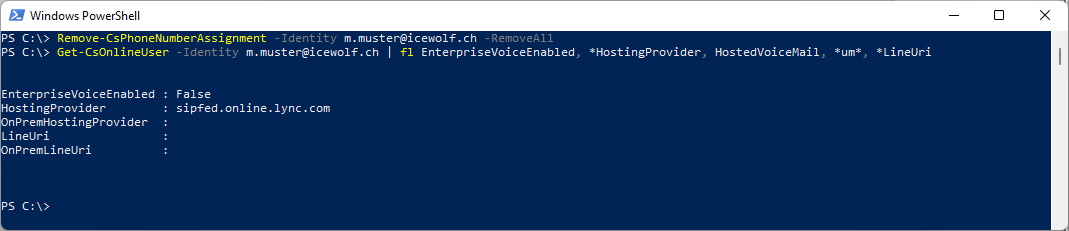
It's good Practice to also remove the TeamsPhone (PhoneSystem / MCOEV ) License
###############################################################################
# Remove PhoneSystem / MCOEV License
###############################################################################
Set-MgUserLicense -UserId m.muster@icewolf.ch -AddLicenses @() -RemoveLicenses @('e43b5b99-8dfb-405f-9987-dc307f34bcbd') #PhoneSystem / MCOEV
# Remove PhoneSystem / MCOEV License
###############################################################################
Set-MgUserLicense -UserId m.muster@icewolf.ch -AddLicenses @() -RemoveLicenses @('e43b5b99-8dfb-405f-9987-dc307f34bcbd') #PhoneSystem / MCOEV

If you don't wait long enough, between the Assignment of the PhoneSystem License and Set-MgUserLicense you will get this Error:
Code: BadRequest
Message:The user '<ID' already has a telephone number set in the on-premises Active Directroy and synchronized...

I did write a Function to set and Remove the TeamsPhone Number including adding and removing the PhoneSystem License.
You can find the Code in my GitHub Repo
Connect-MicrosoftTeams
Connect-MgGraph -Scopes User.ReadWrite.All
Set-TeamsPhoneNumber -UPN m.muster@icewolf.ch -PhoneNumber "+41215553975" -PhoneNumberType "DirectRouting"
Connect-MgGraph -Scopes User.ReadWrite.All
Set-TeamsPhoneNumber -UPN m.muster@icewolf.ch -PhoneNumber "+41215553975" -PhoneNumberType "DirectRouting"
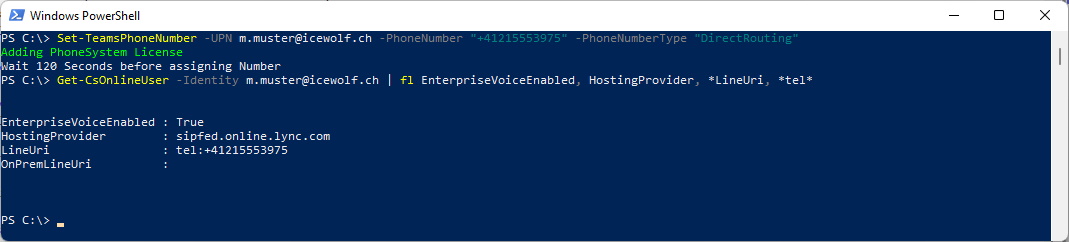
Connect-MicrosoftTeams
Connect-MgGraph -Scopes User.ReadWrite.All
Remove-TeamsPhoneNumber -UPN m.muster@icewolf.ch
Connect-MgGraph -Scopes User.ReadWrite.All
Remove-TeamsPhoneNumber -UPN m.muster@icewolf.ch
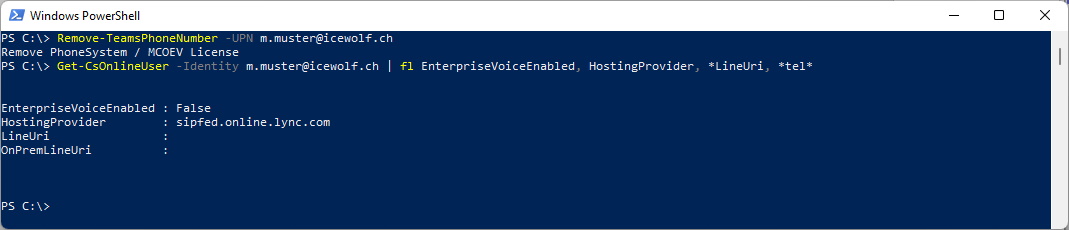
Function Set-TeamsPhoneNumber
{
Param(
[Parameter(Mandatory=$true)][String]$UPN,
[Parameter(Mandatory=$true)][String]$PhoneNumber,
[Parameter(Mandatory=$true)][String]$PhoneNumberType
)
#Check Licenses of User
$Licenses = Get-MgUserLicenseDetail -UserId $UPN
#Check Teams License
$TeamsLicense = $Licenses | where {$_.ServicePlans.ServicePlanname -match "TEAMS1"}
If ($Null -eq $TeamsLicense)
{
Throw "User has no Teams License"
}
#Check PhoneSystem
$PhoneSystemLicense = $Licenses | where {$_.SkuId -eq "e43b5b99-8dfb-405f-9987-dc307f34bcbd"} #PhoneSystem / MCOEV
If ($Null -eq $PhoneSystemLicense)
{
Write-Host "Adding PhoneSystem License" -ForeGroundColor Green
Set-MgUserLicense -UserId $UPN -AddLicenses @{SkuId = 'e43b5b99-8dfb-405f-9987-dc307f34bcbd'} -RemoveLicenses @() | Out-Null #PhoneSystem / MCOEV
} else {
Write-Host "PhoneSystem License found"
[Bool]$NoWait = $true
}
Do
{
$Licenses = Get-MgUserLicenseDetail -UserId $UPN
$PhoneSystemLicense = $Licenses | where {$_.SkuId -eq "e43b5b99-8dfb-405f-9987-dc307f34bcbd"} #PhoneSystem / MCOEV
If ($Null -eq $PhoneSystemLicense)
{
Write-Host "No PhoneSystem License found" -ForeGroundColor Yellow
}
Start-Sleep -Seconds 5
} while ($Null -eq $PhoneSystemLicense)
If ($NoWait -eq $true)
{
#Do Nothing
} else {
Write-Host "Wait 120 Seconds before assigning Number"
Start-Sleep -Seconds 120
}
###############################################################################
# Set-CsPhoneNumberAssignment
# https://docs.microsoft.com/en-us/powershell/module/teams/set-csphonenumberassignment?view=teams-ps
###############################################################################
Set-CsPhoneNumberAssignment -Identity $UPN -PhoneNumber $PhoneNumber -PhoneNumberType $PhoneNumberType
}
Function Remove-TeamsPhoneNumber
{
Param(
[Parameter(Mandatory=$true)][String]$UPN
)
$CsUser = Get-CsOnlineUser -Identity m.muster@icewolf.ch
If ($CsUser.EnterpriseVoiceEnabled -eq $True)
{
###############################################################################
# Remove-CsPhoneNumberAssignment
# https://docs.microsoft.com/en-us/powershell/module/teams/remove-csphonenumberassignment?view=teams-ps
###############################################################################
Remove-CsPhoneNumberAssignment -Identity $UPN -RemoveAll
#Remove PhoneSystem / MCOEV License
Write-Host "Remove PhoneSystem / MCOEV License"
Set-MgUserLicense -UserId $UPN -AddLicenses @() -RemoveLicenses @('e43b5b99-8dfb-405f-9987-dc307f34bcbd') | Out-Null
} else {
Write-Host "EnterpriseVoiceEnabled is already False"
}
}
{
Param(
[Parameter(Mandatory=$true)][String]$UPN,
[Parameter(Mandatory=$true)][String]$PhoneNumber,
[Parameter(Mandatory=$true)][String]$PhoneNumberType
)
#Check Licenses of User
$Licenses = Get-MgUserLicenseDetail -UserId $UPN
#Check Teams License
$TeamsLicense = $Licenses | where {$_.ServicePlans.ServicePlanname -match "TEAMS1"}
If ($Null -eq $TeamsLicense)
{
Throw "User has no Teams License"
}
#Check PhoneSystem
$PhoneSystemLicense = $Licenses | where {$_.SkuId -eq "e43b5b99-8dfb-405f-9987-dc307f34bcbd"} #PhoneSystem / MCOEV
If ($Null -eq $PhoneSystemLicense)
{
Write-Host "Adding PhoneSystem License" -ForeGroundColor Green
Set-MgUserLicense -UserId $UPN -AddLicenses @{SkuId = 'e43b5b99-8dfb-405f-9987-dc307f34bcbd'} -RemoveLicenses @() | Out-Null #PhoneSystem / MCOEV
} else {
Write-Host "PhoneSystem License found"
[Bool]$NoWait = $true
}
Do
{
$Licenses = Get-MgUserLicenseDetail -UserId $UPN
$PhoneSystemLicense = $Licenses | where {$_.SkuId -eq "e43b5b99-8dfb-405f-9987-dc307f34bcbd"} #PhoneSystem / MCOEV
If ($Null -eq $PhoneSystemLicense)
{
Write-Host "No PhoneSystem License found" -ForeGroundColor Yellow
}
Start-Sleep -Seconds 5
} while ($Null -eq $PhoneSystemLicense)
If ($NoWait -eq $true)
{
#Do Nothing
} else {
Write-Host "Wait 120 Seconds before assigning Number"
Start-Sleep -Seconds 120
}
###############################################################################
# Set-CsPhoneNumberAssignment
# https://docs.microsoft.com/en-us/powershell/module/teams/set-csphonenumberassignment?view=teams-ps
###############################################################################
Set-CsPhoneNumberAssignment -Identity $UPN -PhoneNumber $PhoneNumber -PhoneNumberType $PhoneNumberType
}
Function Remove-TeamsPhoneNumber
{
Param(
[Parameter(Mandatory=$true)][String]$UPN
)
$CsUser = Get-CsOnlineUser -Identity m.muster@icewolf.ch
If ($CsUser.EnterpriseVoiceEnabled -eq $True)
{
###############################################################################
# Remove-CsPhoneNumberAssignment
# https://docs.microsoft.com/en-us/powershell/module/teams/remove-csphonenumberassignment?view=teams-ps
###############################################################################
Remove-CsPhoneNumberAssignment -Identity $UPN -RemoveAll
#Remove PhoneSystem / MCOEV License
Write-Host "Remove PhoneSystem / MCOEV License"
Set-MgUserLicense -UserId $UPN -AddLicenses @() -RemoveLicenses @('e43b5b99-8dfb-405f-9987-dc307f34bcbd') | Out-Null
} else {
Write-Host "EnterpriseVoiceEnabled is already False"
}
}
###############################################################################
# Main Script
###############################################################################
Connect-MicrosoftTeams
Connect-MgGraph -Scopes User.ReadWrite.All
Set-TeamsPhoneNumber -UPN m.muster@icewolf.ch -PhoneNumber "+41215553975" -PhoneNumberType "DirectRouting"
Get-CsOnlineUser -Identity m.muster@icewolf.ch | fl EnterpriseVoiceEnabled, HostingProvider, *LineUri, *tel*
Remove-TeamsPhoneNumber -UPN m.muster@icewolf.ch
# Main Script
###############################################################################
Connect-MicrosoftTeams
Connect-MgGraph -Scopes User.ReadWrite.All
Set-TeamsPhoneNumber -UPN m.muster@icewolf.ch -PhoneNumber "+41215553975" -PhoneNumberType "DirectRouting"
Get-CsOnlineUser -Identity m.muster@icewolf.ch | fl EnterpriseVoiceEnabled, HostingProvider, *LineUri, *tel*
Remove-TeamsPhoneNumber -UPN m.muster@icewolf.ch
Regards
Andres Bohren
