Use Azure Storage Queue with PowerShell
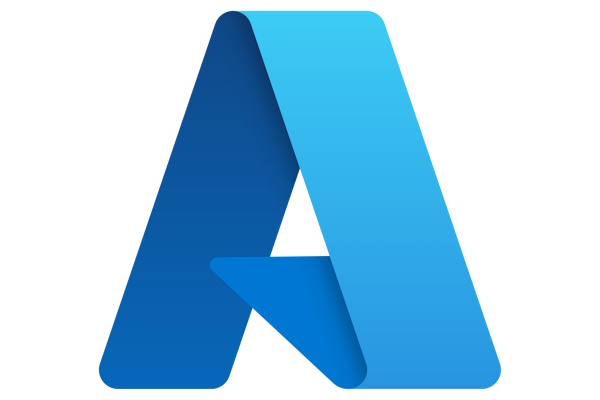
Hi All,
Last year i’ve created the Swiss Domain Security Report Q4 2023 where i ran a PowerShell 7 Script with up to 15 paralell Processes to analize the 2.5 Mio .ch Domains on a Virtual Machine on my Homelab.
For this Year i am thinking about crating a more Cloud native approach with an Azure Function with a Queue storage trigger
Storage Queue
For that i needed to create an Azure Storage Queue
View the Storage Queue with Azure Storage Explorer
PowerShell
We need the AZ PowerShell Modules to connect with Azure
The whole script is also published at my GitHub Repo
#Connect to Azure
Write-Host "Connect to Azure"
Connect-AzAccount -TenantId 46bbad84-29f0-4e03-8d34-f6841a5071ad -Subscription 42ecead4-eae9-4456-997c-1580c58b54ba
Get the Storage Queue
#Get Storage Queue
$Storagequeue = Get-AzStorageAccount -Name devicewolf -ResourceGroupName RG_DEV | Get-AzStorageQueue -Name demoqueue
$Storagequeue
The interesting thing is that the Message has to be Base64 Encoded
#Add Message to Queue
$Message = "icewolf.ch"
$Bytes = [System.Text.Encoding]::ASCII.GetBytes($Message)
$MessageBase64 =[Convert]::ToBase64String($Bytes)
$Storagequeue.QueueClient.SendMessageAsync($MessageBase64)
Now we can see the Message in Azure Storage Explorer
When reading from a Storage Queue, you need to Decode the Base64 Message
#Get Message from Queue
# Set the amount of time you want to entry to be invisible after read from the queue
# If it is not deleted by the end of this time, it will show up in the queue again
$visibilityTimeout = [System.TimeSpan]::FromSeconds(10)
$queueMessage = $Storagequeue.QueueClient.ReceiveMessage($visibilityTimeout)
$EncodedMessage = $queueMessage.Value.MessageText
$Message = [System.Text.Encoding]::ASCII.GetString([System.Convert]::FromBase64String($EncodedMessage))
Write-Host "Message: $Message"
Here we delete the Message from the Storage Queue
# Receive one message from the queue, then delete the message.
$visibilityTimeout = [System.TimeSpan]::FromSeconds(10)
$queueMessage = $Storagequeue.QueueClient.ReceiveMessage($visibilityTimeout)
$Storagequeue.QueueClient.DeleteMessage($queueMessage.Value.MessageId, $queueMessage.Value.PopReceipt)
After the Message has been deleted, the Storage Queue is empty again
Additional Information:
Regards
Andres Bohren